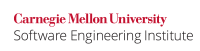
Java input classes such as Scanner
and BufferedInputStream
facilitate fast, non-blocking I/O by buffering an underlying input stream. Programs can create multiple wrappers on an InputStream
. Programs that use multiple wrappers around a single stream, however, can behave unpredictably depending on whether the wrappers allow look-ahead. An attacker can exploit this difference in behavior by, for example, redirecting System.in
(from a file) or by using the System.setIn()
method to redirect System.in
. In general, any input stream that supports non-blocking buffered I/O is susceptible to this form of misuse.
An input stream must not have more than one buffered wrapper. Instead, create and use only one wrapper per input stream, either by passing it as an argument to the methods that need it or by declaring it as a class variable.
Noncompliant Code Example
This noncompliant code example creates multiple BufferedInputStream
wrappers on System.in
, even though there is only one declaration of a BufferedInputStream
. The getChar()
method creates a new BufferedInputStream
each time it is called. Data that are read from the underlying stream and placed in the buffer during execution of one call cannot be replaced in the underlying stream so that a second call has access to them. Consequently, data that remain in the buffer at the end of a particular execution of getChar()
are lost. Although this noncompliant code example uses a BufferedInputStream
, any buffered wrapper is unsafe; this condition is also exploitable when using a Scanner
, for example.
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // Down casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream("input.dat")); System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); // foward to handler } } }
Implementation Details (POSIX)
This program was compiled under Java 1.6.0. When run from the command line, the program successfully takes two characters as input and prints them out. However, when run with a file redirected to standard input, the program throws an EOFException
because the second call to getChar()
finds no characters to read upon encountering the end of the stream.
It may appear that the mark()
and reset()
methods of BufferedInputStream
could be used to replace the read bytes. However, these methods provide look-ahead by operating on the internal buffers of the BufferedInputStream
rather than by operating directly on the underlying stream. Because the example code creates a new BufferedInputStream
on each call to getchar()
, the internal buffers of the previous BufferedInputStream
are lost.
Compliant Solution (Class Variable)
Create and use only a single BufferedInputStream
on System.in
. This compliant solution ensures that all methods can access the BufferedInputStream
by declaring it as a class variable.
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is to advance to the next line // The noncompliant code example deceptively // appeared to work without it (in some cases) return (char)input; } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } }
Implementation Details (POSIX)
This program was compiled under Java 1.6.0. When run from the command line, the program successfully takes two characters as input and prints them out. Unlike the NCCE, this program also produces correct output when run with a file redirected to standard input.
Compliant Solution (Accessible Class Variable)
If a program intends to use System.in
as well as this class InputLibrary
, the program must use the same buffered wrapper as does this class, rather than creating and using its own additional buffered wrapper. Consequently library InputLibrary
must make available a reference to the buffered wrapper to support this functionality.
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); static BufferedInputStream getBufferedWrapper() { return in; } // ...other methods } // Some code that requires user input from System.in class AppCode { private static BufferedInputStream in; AppCode() { in = InputLibrary.getBufferedWrapper(); } // ...other methods }
Note that reading from a stream is not a thread-safe operation by default; consequently, this scheme may not work well in multi-threaded environments. In such cases, explicit synchronization is required.
Risk Assessment
Creating multiple buffered wrappers around an InputStream
can cause unexpected program behavior when the InputStream
is re-directed.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO05-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
Sound automated detection of this vulnerability is not feasible in the general case. Heuristic approaches may be useful.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="d0fe83b1-92ad-4581-8ca0-6981ba2a8008"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
[method read |
http://java.sun.com/javase/6/docs/api/java/io/InputStream.html#read()] |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="3bd4a36c-b484-44e3-9bd6-74bc214a4d8b"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
[class BufferedInputStream |
http://java.sun.com/javase/6/docs/api/java/io/BufferedInputStream.html] |
]]></ac:plain-text-body></ac:structured-macro> |
void FIO04-J. Do not operate on files in shared directories 12. Input Output (FIO) FIO06-J. Close resources when they are no longer needed