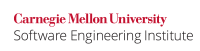
The usual way of initializing an object is to use a constructor. Sometimes it is required to limit the number of instances of the sub-object to just one (this is similar to a singleton, however, the sub-object may or may not be static
). In addition, a technique called lazy initialization is used to defer the construction of the sub-object until it is actually required. Reasons for doing so include optimization and breaking harmful circularities in class and instance initialization [[Bloch 05]].
For these purposes, instead of a constructor, a class or an instance method should be used for initialization, depending on whether the sub-object is static
or not. The method checks whether the instance has already been created and if not, creates it. If the instance already exists, it simply returns it. This is shown below:
// Correct single threaded version using lazy initialization class Foo { private Helper helper = null; public Helper getHelper() { if (helper == null) { helper = new Helper(); } return helper; } // ... }
In a multi-threading scenario, the initialization must be synchronized so that two or more threads do not create multiple instances of the sub-object. The code shown below is safe for execution in a multithreaded environment, albeit slower than the previous, single threaded code example.
// Correct multithreaded version using synchronization class Foo { private Helper helper = null; public synchronized Helper getHelper() { if (helper == null) { helper = new Helper(); } return helper; } // ... }
The double checked locking (DCL) idiom is sometimes used to provide lazy initialization in multithreaded code. In a multi-threading scenario, lazy initialization is supplemented by reducing the cost of synchronization on each method access by limiting the synchronization to the case where the instance is to be created and forgoing it when retrieving an already created instance.
The double-checked locking pattern eliminates method synchronization and uses block synchronization. It strives to make the previous code example faster by installing a null
check before attempting to synchronize. This makes expensive synchronization necessary only for initialization, and dispensable for the common case of retrieving the value. The noncompliant code example shows the originally proposed DCL pattern.
Noncompliant Code Example
This noncompliant code example uses the incorrect form of the double checked locking idiom.
// "Double-Checked Locking" idiom class Foo { private Helper helper = null; public Helper getHelper() { if (helper == null) { synchronized(this) { if (helper == null) { helper = new Helper(); } } } return helper; } // other functions and members... }
According to the Java Memory Model (discussion reference) [[Pugh 04]]:
... writes that initialize the
Helper
object and the write to thehelper
field can be done or perceived out of order. As a result, a thread which invokesgetHelper()
could see a non-null reference to ahelper
object, but see the default values for fields of thehelper
object, rather than the values set in the constructor.Even if the compiler does not reorder those writes, on a multiprocessor the processor or the memory system may reorder those writes, as perceived by a thread running on another processor.
This makes the originally proposed double-checked locking pattern insecure. The rule CON26-J. Do not publish partially-constructed objects discusses further the possibility of a non-null reference to a helper
object that observes default values for fields in the helper
object.
Compliant Solution (volatile
)
This compliant solution declares the Helper
object as volatile
and consequently, uses the correct form of the double-check locking idiom.
// Works with acquire/release semantics for volatile // Broken under JDK 1.4 and earlier class Foo { private volatile Helper helper = null; public Helper getHelper() { if (helper == null) { synchronized(this) { if (helper == null) { helper = new Helper(); // If the helper is null, create a new instance } } } return helper; // If helper is non-null, return its instance } }
JDK 5.0 allows a write of a volatile
variable to be reordered with respect to a previous read or write. A read of a volatile
variable cannot be reordered with respect to any following read or write. Because of this, the double checked locking idiom can work when helper
is declared volatile
. If a thread initializes the Helper
object, a [happens-before relationship] is established between this thread and another that retrieves and returns the instance. [[Pugh 04]] and [[Manson 04]]
"Today, the double-check idiom is the technique of choice for lazily initializing an instance field. While you can apply the double-check idiom to static
fields as well, there is no reason to do so: the lazy initialization holder class idiom is a better choice." [[Bloch 08]].
Compliant Solution (immutable
)
In this solution the Foo
class is unchanged, but the Helper
class is immutable. In this case, the Helper
class is guaranteed to be fully constructed before becoming visible. The object must be truly immutable; it is not sufficient for the program to refrain from modifying the object.
public class Helper { private final int n; public Helper(int n) { this.n = n; } // other fields & methods, all fields are final } class Foo { private Helper helper = null; public Helper getHelper() { if (helper == null) { synchronized(this) { if (helper == null) { helper = new Helper(); // If the helper is null, create a new instance } } } return helper; // If helper is non-null, return its instance } }
Note that if Foo
was mutable, the Helper
field would need to be declared volatile
as shown in CON00-J. Declare shared variables as volatile to ensure visibility and limit reordering of accesses. Also, the method getHelper()
is an instance method and the accessibility of the helper
field is private
. This allows safe publication of the Helper
object, in that, a thread cannot observe a partially initialized Foo
object (CON26-J. Do not publish partially-constructed objects).
Compliant Solution (static initialization)
This compliant solution initializes the helper
in the declaration of the static
variable [[Manson 06]].
class Foo { private static final Helper helper = new Helper(42); public static Helper getHelper() {return helper;} }
Variables declared static
are guaranteed to be initialized and made visible to other threads immediately. Static initializers also exhibit these properties. This approach should not be confused with eager initialization because in this case, the Java Language Specification guarantees lazy initialization of the class when it is first used.
Compliant Solution (initialize-on-demand holder class idiom)
This compliant solution explicitly incorporates lazy initialization. It also uses a static
variable as suggested in the previous compliant solution. The variable is declared within a static
inner, Holder
class.
class Foo { static Helper helper; // Lazy initialization private static class Holder { static Helper helper = new Helper(); } public static Helper getInstance() { return Holder.helper; } }
This idiom is called the initialize-on-demand holder class idiom. Initialization of the Holder
class is deferred until the getInstance()
method is called, following which the helper
is initialized. The only limitation of this method is that it works only for static
fields and not instance fields [[Bloch 01]]. This idiom is a better choice than the double checked locking idiom for lazily initializing static
fields [[Bloch 08]].
Compliant Solution (ThreadLocal
storage)
This compliant solution (originally suggested by Alexander Terekhov [[Pugh 04]]) uses a ThreadLocal
object to lazily create a Helper
instance.
class Foo { // If perThreadInstance.get() returns a non-null value, this thread // has done synchronization needed to see initialization of helper private final ThreadLocal perThreadInstance = new ThreadLocal(); private Helper helper = null; public Helper getHelper() { if (perThreadInstance.get() == null) { createHelper(); } return helper; } private final void createHelper() { synchronized(this) { if (helper == null) { helper = new Helper(); } // Any non-null value would do as the argument here perThreadInstance.set(perThreadInstance); } } }
Exceptions
EX1: Explicitly synchronized code (that uses method synchronization or proper block synchronization, that is, enclosing all initialization statements) does not require the use of double-checked locking.
EX2: "Although the double-checked locking idiom cannot be used for references to objects, it can work for 32-bit primitive values (e.g., int's or float's). Note that it does not work for long's or double's, since unsynchronized reads/writes of 64-bit primitives are not guaranteed to be atomic." [[Pugh 04]]. See [CON25-J. Ensure atomicity when reading and writing 64-bit values] for more Information.
Risk Assessment
Using incorrect forms of the double checked locking idiom can lead to synchronization issues.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON22- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[Pugh 04]]
[[Bloch 01]] Item 48: "Synchronize access to shared mutable data"
[[Bloch 08]] Item 71: "Use lazy initialization judiciously"
[[MITRE 09]] CWE ID 609 "Double-Checked Locking"
CON21-J. Facilitate thread reuse by using Thread Pools 11. Concurrency (CON) CON23-J. Address the shortcomings of the Singleton design pattern