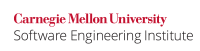
...
Code Block |
---|
namespace MyNamespace { int Ii; } namespace MyNamespace { int Ji; } void f() { MyNamespace::Ii = MyNamespace::Ji = 12; } |
The standard library introduces the namespace std
for standards-provided declarations such as std::string
, std::vector
, and std::for_each
. However, it is undefined behavior to introduce new declarations in namespace std
except under special circumstances. The C++ Standard, [namespace.std], paragraphs 1 and 2 [ISO/IEC 14882-2014], states:
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <functional> #include <iostream> #include <string> class MyString { std::string Datadata; public: MyString(const std::string &Datadata) : Datadata(Datadata) {} const std::string &get_data() const { return Datadata; } }; namespace std { template <> struct plus<string> : binary_function<string, MyString, string> { string operator()(const string &LHSlhs, const MyString &RHSrhs) const { return LHSlhs + RHSrhs.get_data(); } }; } void f() { std::string S1s1("My String"); MyString S2s2(" + Your String"); std::plus<std::string> Pp; std::cout << Pp(S1s1, S2s2) << std::endl; } |
Compliant Solution
...
Code Block | ||||
---|---|---|---|---|
| ||||
#include <iostream> #include <string> class MyString { std::string Datadata; public: MyString(const std::string &Datadata) : Datadata(Datadata) {} const std::string &get_data() const { return Datadata; } }; struct my_plus : std::binary_function<std::string, MyString, std::string> { std::string operator()(const std::string &LHSlhs, const MyString &RHSrhs) const { return LHSlhs + RHSrhs.get_data(); } }; void f() { std::string S1s1("My String"); MyString S2s2(" + Your String"); my_plus Pp; std::cout << Pp(S1s1, S2s2) << std::endl; } |
Risk Assessment
...