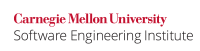
...
Wiki Markup |
---|
The previous compliant solution does not scale very well because a class with several {{synchronized}} methods is a potential bottleneck as far as acquiring locks is concerned and may further lead to contention or deadlock. The class {{ConcurrentHashMap}}, through a more preferable approach, provides several utility methods to perform atomic operations and is used in this compliant solution\[[Lee 09|AA. Java References#Lee 09]\].\[[Lee 09|AA. Java References#Lee 09]\] According to \[[Goetz 06|AA. Java References#Goetz 06]\] section 5.2.1. ConcurrentHashMap: |
ConcurrentHashMap
, along with the other concurrent collections, further improve on the synchronized collection classes by providing iterators that do not throwConcurrentModificationException
, thus eliminating the need to lock the collection during iteration. The iterators returned byConcurrentHashMap
are weakly consistent instead of fail-fast. A weakly consistent iterator can tolerate concurrent modification, traverses elements as they existed when the iterator was constructed, and may (but is not guaranteed to) reflect modifications to the collection after the construction of the iterator.
Code Block | ||
---|---|---|
| ||
public class KeyedCounter { private final ConcurrentMap<String, AtomicInteger> map = new ConcurrentHashMap<String, AtomicInteger>(); public void increment(String key) { AtomicInteger value = new AtomicInteger(0); AtomicInteger old = map.putIfAbsent(key, value); if (old != null) { value = old; } value.incrementAndGet(); // increment the value atomically } public Integer getCount(String key) { AtomicInteger value = map.get(key); return (value == null) ? null : value.get(); } } |
...
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] Class Vector, Class WeakReference \[[JavaThreads 04|AA. Java References#JavaThreads 04]\] 8.2 "Synchronization and Collection Classes" \[[Goetz 06|AA. Java References#Goetz 06]\] 4.4.1. Client-side Locking and, 4.4.2. Composition and 5.2.1. ConcurrentHashMap \[[Lee 09|AA. Java References#Lee 09]\] "Map & Compound Operation" |
...