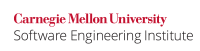
Thread-safety guarantees that no two threads can simultaneously access or modify some shared data. However, if two or more operations need to be performed safely, it becomes necessary to enforce atomicity. It is possible for two threads to read some shared value, independently perform operations on it and induce a race condition while storing the final result. Programmers usually assume that a thread-safe Collection
does not require explicit synchronization which can be a misleading practice. It follows that a thread-safe Collection
may not ensure program correctness.
Noncompliant code Example
This noncompliant example is comprised of an ArrayList
collection which is non-thread-safe by default. There is, however, a way around this drawback. Most thread-unsafe classes have a synchronized thread-safe version, for example, Collections.synchronizedList
is a good substitute for ArrayList
and Collections.synchronizedMap
is a good alternative to HashMap
. One pitfall described in the coming lines, remains to be addressed even when the particular Collection
offers thread-safety benefits.
The operations within a thread's run()
method are non-atomic. That is, it is possible for the first thread to operate on data that it does not expect. The superfluous data may be fed in by other threads whilst the first thread is still executing. Conversely, as the toArray()
method produces a copy of the parameter, it is possible that the first thread operates on stale data [[JavaThreads 04]]. The code's output, consisting of varying array lengths, signifies a race condition. Such omissions can be pernicious in methods that use complex formulas.
class RaceCollection implements Runnable { private List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public void addIPAddress(InetAddress ia) { synchronized(ips) { ips.add(ia); } } public void removeIPAddress(InetAddress ia) { synchronized(ips) { ips.remove(ia); } } public void nonAtomic() throws InterruptedException { InetAddress[] ia; synchronized(ips) { ia = (InetAddress[]) ips.toArray(new InetAddress[0]); } System.out.println("Number of IPs: " + ia.length); } public void run() { try { addIPAddress(InetAddress.getLocalHost()); nonAtomic(); } catch (UnknownHostException e) { } catch (InterruptedException e) { } } public static void main(String[] args) { RaceCollection rc1 = new RaceCollection(); for(int i=0;i<2;i++) new Thread(rc1).start(); } }
Compliant Solution
To eliminate the race condition, ensure atomicity. This can be achieved by including all statements that use the array list within the synchronized block. This technique is also called client-side locking. [[Goetz 06]]
synchronized(ips) { ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); }
Note that this advice applies to all Collection
classes including the thread-safe Hashtable
class. Enumerations of the objects of a Collection
and iterators also require explicit synchronization on the Collection
object or any single lock object.
Although expensive, CopyOnWriteArrayList
and CopyOnWriteArraySet
classes are sometimes used to create copies of the core Collection
so that iterators do not fail with a runtime exception when some data in the Collection
is modified. These however, suffer from the toArray
dilemma (operating on stale data) described earlier in this rule. Consequently, their use is limited to boosting performance in code where the writes are fewer (or non-existent) as compared to the reads [[JavaThreads 04]]. In all other cases they must be avoided.
Compliant Solution
Composition offers more benefits as compared to the previous solution, although at the cost of a slight performance penalty (refer to [OBJ01-J. Understand how a superclass can affect a subclass] for details on how to implement composition). This allows the CompositeCollection
class to use its own intrinsic lock in a way that is completely independent of the lock of the underlying list class. This provides consistent locking even when the underlying list is not thread-safe or when it changes its locking policy. [[Goetz 06]]
class CompositeCollection implements Runnable { private List<InetAddress> ips = Collections.synchronizedList(new ArrayList<InetAddress>()); public CompositeCollection(List<InetAddress> list) { this.ips = list; } public synchronized void addIPAddress(InetAddress ia) { ips.add(ia); } /* other methods */ public synchronized void atomic() throws InterruptedException { InetAddress[] ia; ia = (InetAddress[]) ips.toArray(new InetAddress[0]); System.out.println("Number of IPs: " + ia.length); } }
Yet another method is to extend the base class and synchronize on the method that is desired to be atomic, however, it is not recommended because it goes against the spirit of limiting class extension ([OBJ33-J. Limit the extensibility of non-final classes and methods to only trusted subclasses]). Moreover, Goetz et al. [[Goetz 06]] cite other reasons:
Extension is more fragile than adding code directly to a class, because the implementation of the synchronization policy is now distributed over multiple, separately maintained source files. If the underlying class were to change its synchronization policy by choosing a different lock to guard its state variables, the subclass would subtly and silently break, because it no longer used the right lock to control concurrent access to the base class's state.
Noncompliant Code Example
This noncompliant code example defines a thread-unsafe KeyedCounter
class. Even though the HashMap
field is synchronized, the overall increment
operation is not atomic. [[Lee 09]]
public class KeyedCounter { private Map<String,Integer> map = Collections.synchronizedMap(new HashMap<String,Integer>()); public void increment(String key) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue()+1; map.put(key, value); } public Integer getCount(String key) { return map.get(key); } }
Compliant Solution
This compliant solution declares the increment()
method as synchronized
to ensure atomicity. [[Lee 09]]
public class KeyedCounter { private Map<String,Integer> map = new HashMap<String,Integer>(); public synchronized void increment(String key) { Integer old = map.get(key); int value = (old == null) ? 1 : old.intValue()+1; map.put(key, value); } public synchronized Integer getCount(String key) { return map.get(key); } }
Compliant Solution
The previous compliant solution does not scale very well because a class with several synchronized
methods is a potential bottleneck as far as acquiring locks is concerned and may further lead to contention or deadlock. The class ConcurrentHashMap
, through a more preferable approach, provides several utility methods to perform atomic operations and is used in this compliant solution[[Lee 09]]. According to [[Goetz 06]] section 5.2.1. ConcurrentHashMap:
ConcurrentHashMap
, along with the other concurrent collections, further improve on the synchronized collection classes by providing iterators that do not throwConcurrentModificationException
, thus eliminating the need to lock the collection during iteration. The iterators returned byConcurrentHashMap
are weakly consistent instead of fail-fast. A weakly consistent iterator can tolerate concurrent modification, traverses elements as they existed when the iterator was constructed, and may (but is not guaranteed to) reflect modifications to the collection after the construction of the iterator.
public class KeyedCounter { private final ConcurrentMap<String, AtomicInteger> map = new ConcurrentHashMap<String, AtomicInteger>(); public void increment(String key) { AtomicInteger value = new AtomicInteger(0); AtomicInteger old = map.putIfAbsent(key, value); if (old != null) { value = old; } value.incrementAndGet(); // increment the value atomically } public Integer getCount(String key) { AtomicInteger value = map.get(key); return (value == null) ? null : value.get(); } }
Risk Assessment
Non-atomic code can induce race conditions and affect program correctness.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON38- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Vector, Class WeakReference
[[JavaThreads 04]] 8.2 "Synchronization and Collection Classes"
[[Goetz 06]] 4.4.1. Client-side Locking, 4.4.2. Composition and 5.2.1. ConcurrentHashMap
[[Lee 09]] "Map & Compound Operation"
CON37-J. Never apply a lock to methods making network calls 10. Concurrency (CON) CON39-J. Ensure atomicity of 64-bit operations