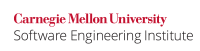
_Lazy initialization_ defers the construction of a member field or an object referred to by a member field until an instance is actually required rather than computing the field value or constructing the referenced object in the class's constructor. Lazy initialization helps to break harmful circularities in class and instance initialization . It also enables other optimizations \[ [Bloch 2005|AA. References#Bloch 05]\]. Wiki Markup
Lazy initialization uses either a class or an instance method, depending on whether the member object is static. The method checks whether the instance has already been created and, if not, creates it. When the instance already exists, the method simply returns the instance:
...
Code Block | ||
---|---|---|
| ||
// "Double-Checked Locking" idiom final class Foo { private Helper helper = null; public Helper getHelper() { if (helper == null) { synchronized (this) { if (helper == null) { helper = new Helper(); } } } return helper; } // Other methods and members... } |
According to Pugh \[ [Pugh 2004|AA. References#Pugh 04]\] Wiki Markup
Writes that initialize the
Helper
object and the write to thehelper
field can be done or perceived out of order. As a result, a thread which invokesgetHelper()
could see a non-null reference to ahelper
object, but see the default values for fields of thehelper
object, rather than the values set in the constructor.Even if the compiler does not reorder those writes, on a multiprocessor, the processor or the memory system may reorder those writes, as perceived by a thread running on another processor.
...
Code Block | ||
---|---|---|
| ||
// Works with acquire/release semantics for volatile // Broken under JDK 1.4 and earlier final class Foo { private volatile Helper helper = null; public Helper getHelper() { if (helper == null) { synchronized (this) { if (helper == null) { helper = new Helper(); } } } return helper; } } |
...
When a thread initializes the {{Helper
}} object, a [happens-before relationship|BB. Glossary#happens-before order] is established between this thread and any other thread that retrieves and returns the instance \[ [Pugh 2004|AA. References#Pugh 04], [Manson 2004|AA. References#Manson 04]\].
Compliant Solution (Static Initialization)
This compliant solution initializes the {{ Wiki Markup helper
}} field in the declaration of the static variable \[ [Manson 2006|AA. References#Manson 06]\].
Code Block | ||
---|---|---|
| ||
final class Foo { private static final Helper helper = new Helper(); public static Helper getHelper() { return helper; } } |
...
Code Block | ||
---|---|---|
| ||
final class Foo { // Lazy initialization private static class Holder { static Helper helper = new Helper(); } public static Helper getInstance() { return Holder.helper; } } |
...
Initialization of the static {{helper
}} field is deferred until the {{getInstance()
}} method is called. The necessary happens-before relationships are created by the combination of the class loader's actions loading and initializing the {{Holder
}} instance and the guarantees provided by the Java memory model. This idiom is a better choice than the double-checked locking idiom for lazily initializing static fields \[ [Bloch 2008|AA. References#Bloch 08]\]. However, this idiom cannot be used to lazily initialize instance fields \[ [Bloch 2001|AA. References#Bloch 01]\].
Compliant Solution (ThreadLocal
Storage)
...
This compliant solution (originally suggested by Alexander Terekhov \[ [Pugh 2004|AA. References#Pugh 04]\]) uses a {{ThreadLocal
}} object to track whether each individual thread has participated in the synchronization that creates the needed happens-before relationships. Each thread stores a non-null value into its thread-local {{perThreadInstance
}} only inside the synchronized {{createHelper()
}} method; consequently, any thread that sees a null value must establish the necessary happens-before relationships by invoking {{createHelper()
}}.
Code Block | ||
---|---|---|
| ||
final class Foo { private final ThreadLocal<Foo> perThreadInstance = new ThreadLocal<Foo>(); private Helper helper = null; public Helper getHelper() { if (perThreadInstance.get() == null) { createHelper(); } return helper; } private synchronized void createHelper() { if (helper == null) { helper = new Helper(); } // Any non-null value can be used as an argument to set() perThreadInstance.set(this); } } |
...
Code Block | ||
---|---|---|
| ||
public final class Helper { private final int n; public Helper(int n) { this.n = n; } // Other fields and methods, all fields are final } final class Foo { private Helper helper = null; public Helper getHelper() { if (helper == null) { synchronized (this) { if (helper == null) { helper = new Helper(42); } } } return helper; } } |
Exceptions
...
*LCK10-EX0:* Use of the noncompliant form of the double-checked locking idiom is permitted for 32-bit primitive values (for example, {{int
}} or {{float
}}) \[ [Pugh 2004|AA. References#Pugh 04]\], although this usage is discouraged. The noncompliant form establishes the necessary happens-before relationship between threads that see an initialized version of the primitive value. The second happens-before relationship (for the initialization of the fields of the referent) is of no practical value because unsynchronized reads and writes of primitive values up to 32-bits are guaranteed to be atomic. Consequently, the noncompliant form establishes the only needed happens-before relationship in this case. Note, however, that the noncompliant form fails for {{long
}} or {{double
}} because unsynchronized reads or writes of 64-bit primitives lack a guarantee of atomicity and consequently require a second happens-before relationship to guarantee that all threads see only fully assigned 64-bit values (See rule [VNA05-J. Ensure atomicity when reading and writing 64-bit values] for more information.)
Risk Assessment
Using incorrect forms of the double-checked locking idiom can lead to synchronization problems and can expose partially initialized objects.
...
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="09d3bf18-8476-46a4-bb33-9de4309de337"><ac:plain-text-body><![CDATA[ | [ [API 2006AA. References#API 06]] |
| ]]></ac:plain-text-body></ac:structured-macro> | <ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="397b3bcc-c9b0-4615-8c8f-7c2b598c39f0"><ac:plain-text-body><![CDATA[ |
[ [Bloch 2001AA. References#Bloch 01] ] | Item 48. Synchronize access to shared mutable data | ]]></ac:plain-text-body></ac:structured-macro> | ||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="caaf0304-d19e-482d-b308-59ac56b0e5b6"><ac:plain-text-body><![CDATA[ | [ [Bloch 2008AA. References#Bloch 08]] | Item 71. Use lazy initialization judiciously]]></ac:plain-text-body></ac:structured-macro><ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="9c9fe6ba-fc38-41a4-befb-2c5e6862aa61"><ac:plain-text-body><![CDATA[ | ||
[ [JLS 2005AA. References#JLS 05]] | §12.4, Initialization of Classes and Interfaces | ]]></ac:plain-text-body></ac:structured-macro> | <ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="40e154b2-cd72-4d11-80bb-cfbd1b71f462"><ac:plain-text-body><![CDATA[ | |
[ [Pugh 2004AA. References#Pugh 04]] | ]]></ac:plain-text-body></ac:structured-macro> |
...