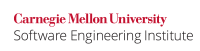
...
Programs must ensure that threads that hold locks on other objects release those locks appropriately before entering the wait state. Additional guidance on waiting and notification is available in THI03-J. Always invoke wait() and await() methods inside a loop and THI02-J. Notify all waiting threads rather than a single thread.
Noncompliant Code Example (Network I/O)
...
Code Block | ||
---|---|---|
| ||
// No synchronization public boolean sendPage(Socket socket, String pageName) { Page targetPage = getPage(pageName); if (targetPage == null){ return false; } return deliverPage(socket, targetPage); } // Requires synchronization private synchronized Page getPage(String pageName) { Page targetPage = null; for (Page p : pageBuff) { if (p.getName().equals(pageName)) { targetPage = p; } } return targetPage; } // Return false if an error occurs, true if successful public boolean deliverPage(Socket socket, Page page) { ObjectOutputStream out = null; boolean result = true; try { // Get the output stream to write the Page to out = new ObjectOutputStream(socket.getOutputStream()); // Send the page to the client out.writeObject(page);out.flush(); } catch (IOException io) { result = false; } finally { if (out != null) { try { out.close(); } catch (IOException e) { result = false; } } } return result; } |
Exceptions
LCK09-J-EX0: Classes that provide an appropriate termination mechanism to callers are permitted to violate this rule (see THI04-J. Ensure that threads performing blocking operations can be terminated).
LCK09-J-EX1: Methods that require multiple locks may hold several locks while waiting for the remaining locks to become available. This constitutes a valid exception, although the programmer must follow other applicable rules, especially LCK07-J. Avoid deadlock by requesting and releasing locks in the same order to avoid deadlock .
...