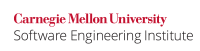
...
This behavior can be result in reading indeterminate values in code that is required to be thread-safe.
Noncompliant Code Example
The Java programming language allows threads to access shared variables. If, in this noncompliant code example, one thread repeatedly calls the method one()
, and another thread repeatedly calls the method two()
:
...
then method two()
could occasionally print a value for i
that is neither zero nor a previous value of j
two()
. A similar problem occurs if i
is declared as a double
.
Compliant Solution (volatile)
This compliant solution declares i
as volatile
. Writes and reads of volatile long
and double
values are always atomic.
Code Block | ||
---|---|---|
| ||
class Test { static volatile long i = 0; static void one(long j) { i = j; } static void two() { System.out.println("i =" + i); } } |
Compliant Solution (atomic variable classes)
This compliant solution uses atomic variable classes from the java.util.concurrent.atomic
package. These classes provide lock-free thread-safe programming on single variables.
Code Block | ||
---|---|---|
| ||
class Test {
static AtomicLong i = 0;
static void one(long j) { i = j; }
static void two() {
System.out.println("i =" + i);
}
}
|
Volatile semantics does guarantee the atomicity of complex operations that involve read-modify-write sequences such as incrementing a value. See CON00-J. Synchronize access to shared mutable variables for more information.
Exceptions
CON25-EX1: If all reads and writes of 64 bit long
and double
values occur within a synchronized method calls, the atomicity of the read/write is guaranteed. This requires that no unsynchronized methods in the class expose the value and that the value is inaccessible (directly or indirectly) from other code.
CON25-EX2: Systems that guarantee that 64 bit long
and double
values are read and written as atomic operations may safely ignore this guideline. It is also possible to hold doubles using the Double.doubleToLongBits
and Double.longBitsToDouble
conversions.
Risk Assessment
Failure to ensure the atomicity of operations involving 64-bit values in multithreaded applications can result in reading and writing indeterminate values.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
CON39 CON25-J | low | probable | medium | P4 | L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] 17.7 Non-atomic Treatment of double and long \[[Goetz 06|AA. Java References#Goetz 06]\] 3.1.2. Nonatomic 64-bit Operations |
...