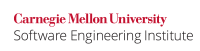
...
The Java programming language allows threads to access shared variables. If, in this noncompliant code example, one thread repeatedly calls the method one()
(but no more than Integer.MAX_VALUE
times in all), and another thread repeatedly calls the method two()
:
...
then method two()
could occasionally print a value for i
that is not one more than the neither zero nor a previous value printed by of j
two()
. A similar problem occurs if i
is declared as a double
.
Compliant Solution (volatile)
This compliant solution declares the variables i
as volatile
. Writes and reads of volatile long
and double
values are always atomic.
Code Block | ||
---|---|---|
| ||
class Test {
static volatile long i = 0;
static void one(long j) { i = j; }
static void two() {
System.out.println("i =" + i);
}
}
|
Compliant Solution (atomic variable classes)
This compliant solution synchronizes calls to methods one()
and two()
. This guarantees these two method calls are treated as atomic operation, including reading and writing to the variable i
uses atomic variable classes from the java.util.concurrent.atomic
package. These classes provide lock-free thread-safe programming on single variables.
Code Block | ||
---|---|---|
| ||
class Test { static longAtomicLong i = 0; static void synchronized one(long j) { i = j; } static synchronized void two() { System.out.println("i =" + i); } } |
Consequently, there is no need to qualify i
as a volatile typeIt is also possible to hold doubles using the Double.doubleToLongBits
and Double.longBitsToDouble
conversions.
Risk Assessment
Failure to ensure the atomicity of operations involving 64-bit values in multithreaded applications can result in reading and writing indeterminate values.
...
Wiki Markup |
---|
\[[JLS 05|AA. Java References#JLS 05]\] 17.7 Non-atomic Treatment of double and long \[[Goetz 06|AA. Java References#Goetz 06]\] 3.1.2. Nonatomic 64-bit Operations |
Wiki Markup |
---|
\[[Goetz 04|AA. Java References#Goetz 04]\] Brian Goetz. Java theory and practice: Going atomic. November 2004. http://www.ibm.com/developerworks/java/library/j-jtp11234/ |
...
CON06-J. Ensure atomicity of thread-safe code 11. Concurrency (CON) CON02-J. Do not use background threads during class initialization