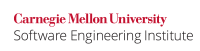
...
Code Block | ||
---|---|---|
| ||
public class SomeObject { // Locks on the object's monitor public synchronized void changeValue() { // ... } public static SomeObject lookup(String name) { // ... } } // Untrusted code String name = // ... SomeObject someObject = new SomeObject.lookup(name); if (someObject == null) { // ... handle error } synchronized (someObject) { while (true) { // Indefinitely delay someObject Thread.sleep(Integer.MAX_VALUE); } } |
The untrusted code attempts to acquire a lock on the object's monitor and, upon succeeding, introduces an indefinite delay that prevents the synchronized changeValue()
method from acquiring the same lock. Furthermore, the object locked is publicly available via the lookup()
method.
Alternatively, an attacker could create a private SomeObject
object and make it available to trusted code to use it before the attacker code grabs and holds the lock.
Note that in the untrusted code, the attacker intentionally violates rule LCK09-J. Do not perform operations that can block while holding a lock.
...