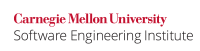
There are two ways to synchronize access to shared mutable variables: method synchronization and block synchronization. Methods declared as synchronized and blocks that synchronize on the this
reference both use the objectâ�€�™s objectâ��‚���„�s monitor (that is, its intrinsic lock). An attacker can manipulate the system to trigger contention and deadlock by obtaining and indefinitely holding the intrinsic lock of an accessible class, consequently causing a denial of service (DoS).
One technique for preventing this vulnerability is the private lock object idiom [Bloch 2001]. This idiom uses the intrinsic lock associated with the instance of a private final java.lang.Object
declared within the class instead of the intrinsic lock of the object itself. This idiom requires the use of synchronized blocks within the classâ�€�™s classâ��‚���„�s methods rather than the use of synchronized methods. Lock contention between the classâ�€�™s classâ��‚���„�s methods and those of a hostile class becomes impossible because the hostile class cannot access the private final lock object.
...
The private lock object idiom is also suitable for classes that are designed for inheritance. When a superclass requests a lock on the objectâ�€�™s objectâ��‚���„�s monitor, a subclass can interfere with its operation. For example, a subclass may use the superclass objectâ�€�™s objectâ��‚���„�s intrinsic lock for performing unrelated operations, causing lock contention and deadlock. Separating the locking strategy of the superclass from that of the subclass ensures that they do not share a common lock and also permits fine-grained locking by supporting the use of multiple lock objects for unrelated operations. This increases the overall responsiveness of the application.
...
When any of these restrictions are violated, the objectâ�€�™s objectâ��‚���„�s intrinsic lock cannot be trusted. But when these restrictions are obeyed, the private lock object idiom fails to add any additional security. Consequently, objects that comply with all of the restrictions are permitted to synchronize using their own intrinsic lock. However, block synchronization using the private lock object idiom is superior to method synchronization for methods that contain nonatomic operations that could either use a more fine-grained locking scheme involving multiple private final lock objects or that lack a requirement for synchronization. Nonatomic operations can be decoupled from those that require synchronization and can be executed outside the synchronized block. Both for this reason and for simplification of maintenance, block synchronization using the private lock object idiom is generally preferred over intrinsic synchronization.
...
The untrusted code attempts to acquire a lock on the objectâ�€�™s objectâ��‚���„�s monitor and, upon succeeding, introduces an indefinite delay that prevents the synchronized changeValue()
method from acquiring the same lock. Note that in the untrusted code, the attacker intentionally violates rule LCK09-J. Do not perform operations that can block while holding a lock.
...
This noncompliant code example locks on a public nonfinal object in an attempt to use a lock other than {{SomeObject}}â�€�™s â��‚���„�s intrinsic lock.
Code Block | ||
---|---|---|
| ||
public class SomeObject { public Object lock = new Object(); public void changeValue() { synchronized (lock) { // ... } } } |
...
Any thread can modify the fieldâ�€�™s fieldâ��‚���„�s value to refer to a different object in the presence of an accessor such as setLock()
. That modification might cause two threads that intend to lock on the same object to lock on different objects, thereby permitting them to execute two critical sections in an unsafe manner. For example, if the lock were changed when one thread was in its critical section, a second thread would lock on the new object instead of the old one and would enter its critical section erroneously.
...
The untrusted code attempts to acquire a lock on the class objectâ�€�™s objectâ��‚���„�s monitor and, upon succeeding, introduces an indefinite delay that prevents the synchronized changeValue()
method from acquiring the same lock.
...