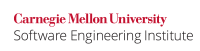
Bit-fields can be used to allow flags or other integer values with small ranges to be packed together to save storage space.
It For bit-fields, it is implementation-defined whether the specifier int
designates the same type as signed int
or the same type as unsigned int
.
Also, C99 requires that "If for bit-fields. According to the C Standard [ISO/IEC 9899:2011], C integer promotions also require that "if an int
can represent all values of the original type (as restricted by the width, for a bit-field), the value is converted to an int
; otherwise, it is converted to an unsigned int
." In the following example:
Code Block |
---|
struct {
unsigned int a: 8;
} bits = {255};
int main(void) {
printf(LANG ", unsigned 8-bit field promotes to %s.\n",
(bits.a << 24) < 0 ? "signed" : "unsigned");
}
|
The type of the expression (bits.a << 24) is compiler dependent and may be either signed or unsigned depending on their interpretation of the standard.
The first interpretation is that when this value is used as an rvalue (e.g., lvalue = rvalue;) the type is "unsigned int" as declared. An unsigned int cannot be represented as an int so integer promotions require that this be an unsigned int, and hence "unsigned".
The second interpretation is that this is an 8-bit integer. As a result, this eight bit value can be represented as an int so integer promotions require that it be converted to int, and hence "signed".
This also has implications for signed long long and unsigned long long types. For example, gcc will also interpret the following as an eight bit value and promote it to int:
This issue is similar to the signedness of plain char
, discussed in INT07-C. Use only explicitly signed or unsigned char type for numeric values. A plain int
bit-field that is treated as unsigned will promote to int
as long as its field width is less than that of int
because int
can hold all values of the original type. This behavior is the same as that of a plain char
treated as unsigned. However, a plain int
bit-field treated as unsigned will promote to unsigned int
if its field width is the same as that of int
. This difference makes a plain int
bit-field even trickier than a plain char
.
Bit-field types other than _Bool
, int
, signed int
, and unsigned int
are implementation-defined. They still obey the integer promotions quoted previously when the specified width is at least as narrow as CHAR_BIT*sizeof(int)
, but wider bit-fields are not portable.
Noncompliant Code Example
This noncompliant code depends on implementation-defined behavior. It prints either -1
or 255
, depending on whether a plain int
bit-field is signed or unsigned.
Code Block | ||||
---|---|---|---|---|
| ||||
struct {
int a: 8;
} bits | ||||
Code Block | ||||
struct { unsigned long long a:8; } ull = {255}; |
<< The following examples need to be completed and tested >>
Non-compliant Code Example 1
In the following non-compliant code example, cBlocks is multiplied by 16 and the result is stored in the unsigned long long int alloc. The result of this multiplication can overflow because it is a 32 bit operation and the resulting value stored in alloc invalid.
Code Block |
---|
struct { unsigned long long size: 24; } ull; void* AllocBlocks(ull cBlocks int main(void) { if (cBlocksprintf("bits.a == 0) return NULL; unsigned long long alloc = cBlocks.size * 16 %d.\n", bits.a); return (alloc < UINT_MAX) ? malloc(cBlocks * 16) : NULL0; } |
Compliant Solution
...
On architectures where unsigned long long int is guaranteed to have 2x the number of bits as size_tupcast the variable used in the multiplication to a 64-bit value. This ensures the multiplication operation is performed
Code Block |
---|
void* AllocBlocks(size_t cBlocks) {
if (cBlocks == 0) return NULL;
unsigned long long alloc =
(unsigned long long)cBlocks.size * 16;
return (alloc < UINT_MAX)
? malloc(cBlocks * 16)
: NULL;
}
|
The assumption concerning the relationship of unsigned long long int and size_t must be document in the header for each file that depends upon this assumption for correct execution.
References
...
This compliant solution uses an unsigned int
bit-field and does not depend on implementation-defined behavior:
Code Block | ||||
---|---|---|---|---|
| ||||
struct {
unsigned int a: 8;
} bits = {255};
int main(void) {
printf("bits.a = %d.\n", bits.a);
return 0;
}
|
Risk Assessment
Making invalid assumptions about the type of a bit-field or its layout can result in unexpected program flow.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT12-C | Low | Unlikely | Medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
Astrée |
| bitfield-type | Fully checked | ||||||
Axivion Bauhaus Suite |
| CertC-INT12 | |||||||
CodeSonar |
| LANG.TYPE.BFSIGN | Bit-field signedness not explicit | ||||||
Compass/ROSE | |||||||||
| CC2.INT12 | Fully implemented | |||||||
Helix QAC |
| C0634, C0635 | |||||||
Klocwork |
| MISRA.BITFIELD.TYPE | |||||||
LDRA tool suite |
| 73 S | Fully implemented | ||||||
Parasoft C/C++test |
| CERT_C-INT12-a | Bit fields shall only be defined to be of type unsigned int or signed int | ||||||
PC-lint Plus |
| 846 | Fully supported | ||||||
Polyspace Bug Finder |
| Checks for bit-field declared without appropriate type (rec. fully covered) | |||||||
RuleChecker |
| bitfield-type | Fully checked | ||||||
SonarQube C/C++ Plugin |
| S814 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
SEI CERT C++ Coding Standard | VOID INT12-CPP. Do not make assumptions about the type of a plain int bit-field when used in an expression |
ISO/IEC TR 24772:2013 | Bit Representations [STR] |
MISRA C:2012 | Rule 10.1 (required) |
Bibliography
[ISO/IEC 9899:2011] | Subclause 6.3.1.1, "Boolean, Characters, and Integers" |
...