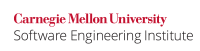
For bit-fields, it is implementation-defined whether the specifier int designates the same type as signed int or the same type as unsigned int.
Also, C99 requires that "If an int can represent all values of the original type, the value is converted to an int; otherwise, it is converted to an unsigned int."
In the following example:
struct { unsigned int a: 8; } bits = {255}; int main(void) { printf(LANG ", unsigned 8-bit field promotes to %s.\n", (bits.a << 24) < 0 ? "signed" : "unsigned"); }
The type of the expression (bits.a << 24) is compiler dependent and may be either signed or unsigned depending on their interpretation of the standard.
The first interpretation is that when this value is used as an rvalue (e.g., lvalue = rvalue;) the type is "unsigned int" as declared. An unsigned int cannot be represented as an int so integer promotions require that this be an unsigned int, and hence "unsigned".
The second interpretation is that this is an 8-bit integer. As a result, this eight bit value can be represented as an int so integer promotions require that it be converted to int, and hence "signed".
This also has implications for signed long long and unsigned long long types. For example, gcc will also interpret the following as an eight bit value and promote it to int:
struct { unsigned long long a:8; } ull = {255};
<< The following examples need to be completed and tested >>
Non-compliant Code Example 1
In the following non-compliant code example, cBlocks is multiplied by 16 and the result is stored in the unsigned long long int alloc. The result of this multiplication can overflow because it is a 32 bit operation and the resulting value stored in alloc invalid.
struct { unsigned long long size: 24; } ull; void* AllocBlocks(ull cBlocks) { if (cBlocks == 0) return NULL; unsigned long long alloc = cBlocks.size * 16; return (alloc < UINT_MAX) ? malloc(cBlocks * 16) : NULL; }
Compliant Solution 1
On architectures where unsigned long long int is guaranteed to have 2x the number of bits as size_tupcast the variable used in the multiplication to a 64-bit value. This ensures the multiplication operation is performed
void* AllocBlocks(size_t cBlocks) { if (cBlocks == 0) return NULL; unsigned long long alloc = (unsigned long long)cBlocks.size * 16; return (alloc < UINT_MAX) ? malloc(cBlocks * 16) : NULL; }
The assumption concerning the relationship of unsigned long long int and size_t must be document in the header for each file that depends upon this assumption for correct execution.
References
- ISO/IEC 9899:1999 6.7.2 Type specifiers