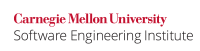
Bit-fields can be used to allow flags or other integer values with small ranges to be packed together to save storage space.
It is implementation-defined whether the specifier int
designates the same type as signed int
or the same type as unsigned int
for bit-fields. C integer promotions also require that "If an int
can represent all values of the original type, the value is converted to an int
; otherwise, it is converted to an unsigned int
."
This is a similar issue to the signedness of plain char
, discussed in INT07-A. Use only explicitly signed or unsigned char type for numeric values. A plain int
bit-field that is treated as unsigned will promote to int
as long as its field width is less than that of int
, because int
can hold all values of the original type. This is the same behavior as that of a plain char
treated as unsigned. However, a plain int
bit-field treated as unsigned will promote to unsigned int
if its field width is the same as that of int
. This difference makes a plain int
bit-field even trickier than a plain char
.
Bit-field types other than _Bool
, int
, signed int
, and unsigned int
are implementation-defined. They still obey the integer promotions quoted above when the specified width is at least as narrow as CHAR_BIT*sizeof(int)
, but wider bit-fields are not portable.
Non-Compliant Code Example
This non-compliant code depends on implementation-defined behavior. It prints either -1 or 255 depending on whether a plain int
bit-field is signed or unsigned.
struct { int a: 8; } bits = {255}; int main(void) { printf("bits.a = %d.\n", bits.a); return 0; }
Compliant Solution
This compliant solution uses an unsigned int
bit-field and does not depend on implementation-defined behavior.
struct { unsigned int a: 8; } bits = {255}; int main(void) { printf("bits.a = %d.\n", bits.a); return 0; }
Risk Assessment
Making invalid assumptions about the type of a bit-field or its layout can result in unexpected program flow.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
INT12-A |
1 (low) |
1 (unlikely) |
2 (medium) |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 6.7.2, "Type specifiers"
[[MISRA 04]] Rule 12.7
INT11-A. Do not make assumptions about the layout of bit-field structures 04. Integers (INT) INT13-A. Do not assume that a right shift operation is implemented as a logical or an arithmetic shift