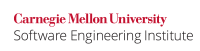
Many existing functions that return an errno
are declared as returning a value of type int
. It is semantically unclear by looking at the function declaration or prototype if these functions return an error status or a value (or worse, some combination of the two).
TR 24731-1 defines a new type of errno_t
which is type int
in <errno.h>
and elsewhere. Many of the functions defined in TR 24731-1 return values of this type. As a matter of programming style, errno_t
should be used as the type of something that deals only with the values that might be found in errno
. For example, a function which returns the value of errno
should be declared as having the return type errno_t
.
Non-Compliant Code Example
int opener(FILE* file, int *width, int *height, int *data_offset) { int file_w; int file_h; int file_o; int offset = 0; if (file == NULL) { return -1; } if (fscanf(file, "%i %i %i", &file_w, &file_h, &file_o) != 3) { return -1; } if (fsetpos(file, &offset) != 0) { return -1; } *width = file_w; *height = file_h; *data_offset = file_o; return 0; }
Compliant Solution
errno_t opener(FILE* file, int *width, int *height, int *data_offset) { int file_w; int file_h; int file_o; int rc; fpos_t offset; if (file == NULL) { return EINVAL; } rc = fgetpos(file, &offset); if (rc != 0) { return (errno_t)rc; } if (fscanf(file, "%i %i %i", &file_w, &file_h, &file_o) != 3) { return EIO; } rc = fsetpos(file, &offset); if (rc != 0) { return -1; } *width = file_w; *height = file_h; *data_offset = file_o; return 0; }
[[ISO/IEC TR 24731-2006]]
[[ISO/IEC 9899-1999:TC2]] Section 6.7.5.3, "Function declarators (including prototypes)"