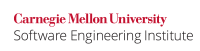
The relational and equality operators are left-associative, not non-associative as they often are in other languages. This allows a C++ programmer to write an expression (particularly an expression used as a condition) that can be easily misinterpreted.
Non-Compliant Code Example
int a = 2; int b = 2; int c = 2; // ... if ( a < b < c ) // condition #1, misleading, likely bug // ... if ( a == b == c ) // condition #2, misleading, likely bug
While the code in the Non-Compliant Code Example compiles correctly, it is unlikely that it means what the author of the code intended. Condition #1 will evaluate to true, rather than false as its author probably intended, and condition #2 will evaluate to false, rather than true as its author probably intended.
Compliant Solution
Treat relational and equality operators as if it were invalid to chain them.
if ( a < b && b < c ) // clearer, and probably what was intended // ... if ( a == b && a == c ) // ditto
Risk Assessment
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP09-A |
1 (low) |
1 (unlikely) |
2 (medium) |
P2 |
L3 |
Other Languages
This rule appears in the C++ Secure Coding Standard as EXP17-CPP. Treat relational and equality operators as if they were nonassociative.
EXP08-A. A switch statement should have a default clause unless every enumeration value is tested 03. Expressions (EXP) EXP30-C. Do not cast away const