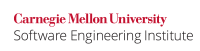
The logical AND and logical OR operators (&&
, ||
) exhibit "short circuit" operation. That is, the second operand is not evaluated if the result can be deduced solely by evaluating the first operand. Consequently, the second operand should not contain side effects because, if it does, it is not apparent whether the side effect occurs.
Non-Compliant Code Example
In this non-compliant code example, the value of i
is incremented only when i >= 0
.
enum { max = 15 }; int i = /* initialize to user supplied value */; if ( (i >= 0 && (i++) <= max) ) { /* code */ }
Although the behavior is well-defined, it is not immediately obvious how this code behaves.
Compliant Solution
This compliant solution exhibits identical behavior but is easier to understand.
enum { max = 15 }; int i = /* initialize to user supplied value */; if (i >= 0) { i++; if (i <= max) { /* code */ } }
Non-Compliant Code Example
In this non-compliant code example, the second operand of the logical OR operator invokes a function that results in side effects.
char *p; if ( p || (p = (char *)malloc(BUF_SIZE)) ) { /* do stuff with p */ } else { /* handle error */ return; }
Compliant Solution
This compliant solution exhibits identical behavior but is easier to understand.
char *p; if (p == NULL) p = (char *)malloc(BUF_SIZE); if (p == NULL) { /* handle error */ return; } /* do stuff with p */
Risk Assessment
Attempting to modify an object that is the second operand to the logical OR or AND operator may cause that object to take on an unexpected value. This can lead to unintended program behavior.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP02-A |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
The LDRA tool suite V 7.6.0 is able to detect violations of this recommendation.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899-1999]] Section 6.5.13, "Logical AND operator," and Section 6.5.14, "Logical OR operator"
EXP01-A. Do not take the size of a pointer to determine the size of the pointed-to type 03. Expressions (EXP) EXP03-A. Do not assume the size of a structure is the sum of the sizes of its members