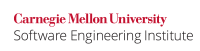
Under many hosted environments it is possible to access the environment through a modified form of main()
:
main(int argc, char *argv[], char *envp[])
According to C99 [[ISO/IEC 9899-1999]]:
In a hosted environment, the main function receives a third argument,
char *envp[]
, that points to a null-terminated array of pointers tochar
, each of which points to a string that provides information about the environment for this execution of the program.
However, modifying the environment by using the setenv()
or putenv()
functions, or by any other means, may cause the environment memory to be reallocated, with the result that envp
now references an incorrect location. For example, [[Austin Group 08]] says the following.
Unanticipated results may occur if
setenv()
changes the external variableenviron
. In particular, if the optionalenvp
argument tomain()
is present, it is not changed, and thus may point to an obsolete copy of the environment (as may any other copy ofenviron
).
When compiled with gcc-3.4.6 and run on Andrew Linux-2.6.16.29, the following code:
extern char **environ; int main(int argc, char *argv[], char *envp[]) { printf("environ: %p\n", environ); printf("envp: %p\n", envp); setenv("MY_NEW_VAR", "new_value", 1); puts("--Added MY_NEW_VAR--"); printf("environ: %p\n", environ); printf("envp: %p\n", envp); }
Yields:
% ./envp-environ environ: 0xbf8656ec envp: 0xbf8656ec --Added MY_NEW_VAR-- environ: 0x804a008 envp: 0xbf8656ec
It is evident from these results that the environment has been relocated as a result of the call to setenv()
.
Non-Compliant Code Example
After a call to setenv()
or other function that modifies the environment, the envp
pointer may no longer reference the environment.
int main(int argc, char *argv[], char *envp[]) { setenv("MY_NEW_VAR", "new_value", 1); if (envp != NULL) { for (size_t i = 0; envp[i] != NULL; i++) { puts(envp[i]); } } return 0; }
Because envp
no longer points to the current environment, this program has undefined behavior.
Compliant Solution (POSIX)
Use environ
in place of envp
when defined.
extern char **environ; int main(int argc, char *argv[]) { setenv("MY_NEW_VAR", "new_value", 1); if (environ != NULL) { for (size_t i = 0; environ[i] != NULL; i++) { puts(environ[i]); } } return 0; }
Note: if you have a great deal of unsafe envp
code, you could save time in your remediation by aliasing. Change:
main(int argc, char *argv[], char *envp[])
To:
extern char **environ; #define envp environ main(int argc, char *argv[])
Risk Assessment
Using the envp
environment pointer after the environment has been modified may result in undefined behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ENV31-C |
1 (low) |
1 (unlikely) |
3 (low) |
P3 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Austin Group 08]] vol. 2, System Interfaces, setenv()
[[ISO/IEC 9899-1999]] Section J.5.1, "Environment Arguments"
ENV30-C. Do not modify the string returned by getenv() 11. Environment (ENV) ENV32-C. Do not call the exit() function more than once