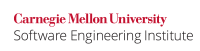
Bit-fields can be used to allow flags or other integer values with small ranges to be packed together to save storage space.
It is implementation-defined whether the specifier int
designates the same type as signed int
or the same type as unsigned int
for bit-fields. According to the C Standard [ISO/IEC 9899:2011], C integer promotions also require that "if an int
can represent all values of the original type (as restricted by the width, for a bit-field), the value is converted to an int
; otherwise, it is converted to an unsigned int
."
This issue is similar to the signedness of plain char
, discussed in INT07-C. Use only explicitly signed or unsigned char type for numeric values. A plain int
bit-field that is treated as unsigned will promote to int
as long as its field width is less than that of int
because int
can hold all values of the original type. This is the same behavior as that of a plain char
treated as unsigned. However, a plain int
bit-field treated as unsigned will promote to unsigned int
if its field width is the same as that of int
. This difference makes a plain int
bit-field even trickier than a plain char
.
Bit-field types other than _Bool
, int
, signed int
, and unsigned int
are implementation-defined. They still obey the integer promotions quoted previously when the specified width is at least as narrow as CHAR_BIT*sizeof(int)
, but wider bit-fields are not portable.
Noncompliant Code Example
This noncompliant code depends on implementation-defined behavior. It prints either -1
or 255
, depending on whether a plain int
bit-field is signed or unsigned.
struct { int a: 8; } bits = {255}; int main(void) { printf("bits.a = %d.\n", bits.a); return 0; }
Compliant Solution
This compliant solution uses an unsigned int
bit-field and does not depend on implementation-defined behavior.
struct { unsigned int a: 8; } bits = {255}; int main(void) { printf("bits.a = %d.\n", bits.a); return 0; }
Risk Assessment
Making invalid assumptions about the type of a bit-field or its layout can result in unexpected program flow.
Recommendation | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
INT12-C | low | unlikely | medium | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Compass/ROSE |
|
|
|
1.2 | bitftype | Fully implemented | |
9.7.1 | 73 S | Fully implemented | |
PRQA QA-C | Unable to render {include} The included page could not be found. | 0634 (I) | Fully implemented |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Guidelines
CERT C++ Secure Coding Standard | INT12-CPP. Do not make assumptions about the type of a plain int bit-field when used in an expression |
---|---|
ISO/IEC TR 24772 | Bit representations [STR] |
MISRA-C | Rule 12.7 (required): Bitwise operators shall not be applied to operands whose underlying type is signed |
Bibliography
[ISO/IEC 9899:2011] | Section 6.3.1.1, "Boolean, Characters, and Integers" |
---|