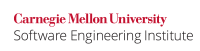
Some environments provide environment pointers that are valid when main()
is called, but may be invalided by operations that modify the environment.
According to C99 [[ISO/IEC 9899:1999]]
In a hosted environment, the main function receives a third argument,
char *envp[]
, that points to a null-terminated array of pointers tochar
, each of which points to a string that provides information about the environment for this execution of the program.
Consequently, under a hosted environments it is possible to access the environment through a modified form of main()
:
main(int argc, char *argv[], char *envp[])
However, modifying the environment by any means may cause the environment memory to be reallocated, with the result that envp
now references an incorrect location.
For example, when compiled with GCC version 3.4.6 and run on a 32-bit Intel GNU/Linux machine, the following code:
extern char **environ; /* ... */ int main(int argc, char const *argv[], char const *envp[]) { printf("environ: %p\n", environ); printf("envp: %p\n", envp); setenv("MY_NEW_VAR", "new_value", 1); puts("--Added MY_NEW_VAR--"); printf("environ: %p\n", environ); printf("envp: %p\n", envp); }
Yields:
% ./envp-environ environ: 0xbf8656ec envp: 0xbf8656ec --Added MY_NEW_VAR-- environ: 0x804a008 envp: 0xbf8656ec
It is evident from these results that the environment has been relocated as a result of the call to setenv()
.
Non-Compliant Code Example (POSIX)
After a call to the POSIX setenv()
function, or other function that modifies the environment, the envp
pointer may no longer reference the environment. POSIX states that [[Open Group 04]]
Unanticipated results may occur if
setenv()
changes the external variableenviron
. In particular, if the optionalenvp
argument tomain()
is present, it is not changed, and as a result may point to an obsolete copy of the environment (as may any other copy ofenviron
).
int main(int argc, char const *argv[], char const *envp[]) { size_t i; if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle Error */ } if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { if (puts(envp[i]) == EOF) { /* Handle Error */ } } } return 0; }
Because envp
no longer points to the current environment, this program has undefined behavior.
Compliant Solution (POSIX)
Use environ
in place of envp
when defined.
extern char **environ; /* ... */ int main(int argc, char const *argv[]) { size_t i; if (setenv("MY_NEW_VAR", "new_value", 1) != 0) { /* Handle Error */ } if (environ != NULL) { for (i = 0; environ[i] != NULL; i++) { if (puts(environ[i]) == EOF) { /* Handle Error */ } } } return 0; }
Non-Compliant Code Example (Windows)
After a call to the Windows _putenv_s()
function, or other function that modifies the environment, the envp
pointer may no longer reference the environment.
According to the Visual C++ reference [[MSDN]]
The environment block passed to
main
andwmain
is a "frozen" copy of the current environment. If you subsequently change the environment via a call toputenv
or_wputenv
, the current environment (as returned bygetenv
/_wgetenv
and the_environ
/_wenviron
variable) will change, but the block pointed to byenvp
will not change.
int main(int argc, char const *argv[], char const *envp[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle Error */ } if (envp != NULL) { for (i = 0; envp[i] != NULL; i++) { if (puts(envp[i]) == EOF) { /* Handle Error */ } } } return 0; }
Because envp
no longer points to the current environment, this program fails to print the value of MY_NEW_VAR
.
Compliant Solution (Windows)
Use _environ
in place of envp
when defined.
_CRTIMP extern char **_environ; /* ... */ int main(int argc, char const *argv[]) { size_t i; if (_putenv_s("MY_NEW_VAR", "new_value") != 0) { /* Handle Error */ } if (_environ != NULL) { for (i = 0; _environ[i] != NULL; i++) { if (puts(_environ[i]) == EOF) { /* Handle Error */ } } } return 0; }
Compliant Solution
Note: if you have a great deal of unsafe envp
code, you can save time in your remediation by aliasing. Change:
int main(int argc, char *argv[], char *envp[]) { /* ... */ }
To:
#if defined (_POSIX_) || defined (__USE_POSIX) extern char **environ; #define envp environ #else _CRTIMP extern char **_environ; #define envp _environ #endif int main(int argc, char *argv[]) { /* ... */ }
Risk Assessment
Using the envp
environment pointer after the environment has been modified may result in undefined behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
ENV31-C |
low |
probable |
medium |
P4 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[ISO/IEC 9899:1999]] Section J.5.1, "Environment Arguments"
[[MSDN]] getenv, _wgetenv
,
_environ, _wenviron
,
_putenv_s, _wputenv_s
[[Open Group 04]] setenv()
ENV30-C. Do not modify the string returned by getenv() 10. Environment (ENV) ENV32-C. atexit handlers must return