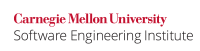
Assertions are a valuable diagnostic tool for finding and eliminating software defects that may result in vulnerabilities (see MSC11-A. Incorporate diagnostic tests using assertions). The runtime assert()
macro has some limitations, however, in that it incurs a runtime overhead and, because it calls abort()
, is only useful for identifying incorrect assumptions and is not intended for runtime error checking. Consequently, runtime assertions are generally unsuitable for server programs or embedded systems.
Static assertion is a new facility in the C++ 0X draft standard [[Becker 08]] and takes the form:
static_assert(constant-expression, string-literal);
According to the C++ 0X draft standard, the constant-expression
in a static assert declaration is a constant expression that can be converted to bool
at compile time. If the value of the converted expression is true, the declaration has no effect. Otherwise the program is ill-formed, and a diagnostic message (which includes the text of the string-literal
) is issued at compile time. For example
/* Passes */ static_assert( sizeof(int) <= sizeof(void*), "sizeof(int) <= sizeof(void*)" ); /* Fails */ static_assert( sizeof(double) <= sizeof(int), "sizeof(double) <= sizeof(int)" );
Static assertion is not available in C99, but the facility is being considered for inclusion in C1X by the ISO/IEC WG14 international standardization working group.
Non-Compliant Code Example
This non-compliant code uses the assert()
macro to assert a property concerning a memory-mapped structure that is essential for the code that uses this structure to behave correctly.
struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; int func(void) { assert(offsetof(timer, DATA) == 4); }
While the use of the runtime assertion is better than nothing, it needs to be placed in a function and executed, typically removed from the actual structure to which it refers. The diagnostic only occurs at runtime, and only if the code path containing the assertion is executed.
Compliant Solution
For assertions involving only constant expressions, some implementations allow the use of a preprocessor conditional statement, as in this example:
struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; #if (offsetof(timer, DATA) != 4) #error "DATA must be at offset 4" #endif
Using #error
directives allows for clear diagnostic messages. Because this approach evaluates assertions at compile time, there is no runtime penalty.
Unfortunately, this solution is not portable. C99 does not require that implementations support sizeof
, offsetof
, or enumeration constants in #if
conditions. According to Section 6.10.1, "Conditional inclusion," all identifiers in the expression that controls conditional inclusion either are or are not macro names. Some compilers allow these constructs in conditionals as an extension, but most do not.
Compliant Solution
This compliant solution mimics the behavior of static_assert
in a portable manner.
#define JOIN(x, y) JOIN_AGAIN(x, y) #define JOIN_AGAIN(x, y) x ## y #define static_assert(e) \ typedef char JOIN(assertion_failed_at_line_, __LINE__) [(e) ? 1 : -1] struct timer { uint8_t MODE; uint32_t DATA; uint32_t COUNT; }; static_assert(offsetof(struct timer, DATA) == 4);
The static_assert()
macro accepts a constant expression e
, which is evaluated as the first operand to the conditional operator. If e
evaluates to nonzero, an array type with a dimension of 1 is defined; otherwise, an array type with a dimension of -1 is defined. Because it is invalid to declare an array with a negative dimension, the resulting type definition will be flagged by the compiler. The name of the array is used to indicate the location of the failed assertion.
The JOIN()
macro used the ##
operator [[ISO/IEC 9899:1999]] to concatenate tokens. See PRE05-C. Understand macro replacement when concatenating tokens or performing stringification to understand how macro replacement behaves in C when using the ##
operator.
Static assertions allow incorrect assumptions to be diagnosed at compile time, instead of resulting in a silent malfunction or runtime error. Because the assertion is performed at compile time, no runtime cost in space or time is incurred. An assertion can be used at file or block scope, and failure results in a meaningful and informative diagnostic error message.
Other uses of static assertion are shown in STR07-A. Use TR 24731 for remediation of existing string manipulation code and FIO35-C. Use feof() and ferror() to detect end-of-file and file errors when sizeof(int) == sizeof(char).
Automated Detection
Compass/ROSE could detect violations of this rule merely by looking for calls to assert()
, and if it is able to evaluate the assertion (due to all values being known at compile time), then the code should use static-assert
instead.
This assumes ROSE can recognize macro invocation.
Risk Assessment
Static assertion is a valuable diagnostic tool for finding and eliminating software defects that may result in vulnerabilities at compile time. The absence of static assertions, however, does not mean that code is incorrect.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL03-A |
low |
unlikely |
high |
P1 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Becker 08]]
[[Eckel 07]]
[[ISO/IEC 9899:1999]] Section 6.10.1, "Conditional inclusion," and Section 6.10.3.3, "The ## operator," and Section 7.2.1, "Program diagnostics"
[[Klarer 04]]
[[Saks 05]]
[[Saks 08]]
DCL02-A. Use visually distinct identifiers 02. Declarations and Initialization (DCL) DCL04-A. Do not declare more than one variable per declaration