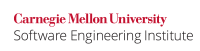
The [[noreturn]]
attribute specifies that a function does not return. The C++ Standard, [dcl.attr.noreturn] paragraph 2 [ISO/IEC 14882-2014], states the following:
If a function
f
is called wheref
was previously declared with thenoreturn
attribute andf
eventually returns, the behavior is undefined.
A function that specifies [[noreturn]]
can prohibit returning by throwing an exception, entering an infinite loop, or calling another function designated with the [[noreturn]]
attribute.
Noncompliant Code Example
In this noncompliant code example, if the value 0
is passed, control will flow off the end of the function, resulting in an implicit return and undefined behavior.
#include <cstdlib> [[noreturn]] void f(int i) { if (i > 0) throw "Received positive input"; else if (i < 0) std::exit(0); }
Compliant Solution
In this compliant solution, the function does not return on any code path.
#include <cstdlib> [[noreturn]] void f(int i) { if (i > 0) throw "Received positive input"; std::exit(0); }
Risk Assessment
Returning from a function marked [[noreturn]]
results in undefined behavior that might be exploited to cause data-integrity violations.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
MSC53-CPP | Medium | Unlikely | Low | P2 | L3 |
Automated Detection
Tool | Version | Checker | Description |
---|---|---|---|
Astrée | 22.10 | invalid-noreturn | Fully checked |
Axivion Bauhaus Suite | 7.2.0 | CertC++-MSC53 | |
Clang | 3.9 | -Winvalid-noreturn | |
CodeSonar | 8.1p0 | LANG.STRUCT.RFNR | Return from noreturn |
Helix QAC | 2024.3 | DF2886 | |
Klocwork | 2024.3 | CERT.MSC.NORETURN_FUNC_RETURNS | |
Parasoft C/C++test | 2023.1 | CERT_CPP-MSC53-a | Never return from functions that should not return |
Polyspace Bug Finder | R2024a | CERT C++: MSC53-CPP | Checks for [[noreturn]] functions returning to caller (rule fully covered) |
PVS-Studio | 7.33 | V1082 | |
RuleChecker | 22.10 | invalid-noreturn | Fully checked |
SonarQube C/C++ Plugin | 4.10 | S935 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Bibliography
[ISO/IEC 14882-2014] | Subclause 7.6.3, " |
5 Comments
Andrzej Kędziora
I would like to propose some improvement in description regarding:
The
[[noreturn]]
attribute specifies that a function does not return.because it could mislead to understand as functions which no return value ("void" functions). I propose to use more precise phrase:
The [[noreturn]] attribute specifies that control flow will not return to the caller.
Similar words I would like to replace here:
"A function that specifies
[[noreturn]]
can prohibit returning by throwing an exception, entering an infinite loop, or calling another function designated with the[[noreturn]]
attribute."using that:
"A function that specifies
[[noreturn]]
can avoid returning to the caller by throwing an exception, entering an infinite loop, or calling another function designated with the[[noreturn]]
attribute."What's your opinion?
BR
Andrzej Kędziora
David Svoboda
The fact that the word "return" here is intransitive (that is, it has no object noun phrase following it) implies that the function neither returns a value, or returns void. So I believe the current wording is correct as is.
Andrzej Kędziora
As you said word "return" could be understood in two ways so for clarity it will be good to emphasize that it's not enough that [[noreturn]] functions will be void. To not break this rule it's necessary that the function can not return to the caller.
David Svoboda
Sorry, no. The verb "return" can be used transitively (to return a value) or intransitively (for void functions that return, but do not return a value). Since its use in any English sentence disambiguates whether it is transitive or intransitive, it can only be understood in one way in its current context.
Andrzej Kędziora
I agree that there is no mistake here in description but it was not clear enough at first glance for me, that's why I proposed improvement.
Anyway, it's good that we at least sort it out here - in comments.