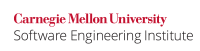
Working with string representations of floating-point values can produce incorrect conclusions about the precision of the values. For example, consider the conversion of a value from type float
to type double
, a widening primitive conversion. Refer to the rule NUM13-J. Beware of precision loss when converting primitive integers to floating-point for more details about such conversions.
String representations of floating-point numbers must not be compared or inspected. When the value of a float
variable must be represented exactly using the double
type, an explicit assignment is more appropriate than first converting the floating-point value to a String
and then to a double
.
Noncompliant Code Example (String Comparison)
This noncompliant code example attempts a string-based comparison of a floating-point number.
int i = 1; String s = Double.valueOf(i / 1000.0).toString(); if (s.equals("0.001")) { // ... }
However s
actually contains the string "0.0010"
. Consequently, the comparison unexpectedly fails.
Noncompliant Code Example (Regex)
This noncompliant code example attempts to mitigate the extra trailing zero by using a regular expression on the string before comparing it.
int i = 1; String s = Double.valueOf(i / 1000.0).toString(); s = s.replaceFirst("[.0]*$", ""); if (s.equals("0.001")) { // ... }
While the comparison does succeed on the code above, it fails on the similar code below, which uses 1/10000.0
instead of 1/1000.0
. The string produced is not 0.00010
but rather 1.0E-4
.
int i = 1; String s = Double.valueOf(i / 10000.0).toString(); s = s.replaceFirst("[.0]*$", ""); if (s.equals("0.0001")) { // ... }
Compliant Solution (String Comparison)
This compliant solution uses the BigDecimal
class to avoid precision loss. It then performs a numeric comparison, which passes as expected.
int i = 1; BigDecimal d = new BigDecimal(Double.valueOf(i / 1000.0).toString()) if (d.compareTo(new BigDecimal("0.001")) == 0) { // ... }
Risk Assessment
Relying on the string representation of floating-point types can result in imprecise values.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
NUM11-J |
low |
likely |
medium |
P6 |
L2 |
Related Vulnerabilities
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="d1181d70-10fd-4639-9230-4dcf5c3fe9a0"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="7d839a55-170e-4692-854e-d0c73448a730"><ac:plain-text-body><![CDATA[ |
[[JLS 2005 |
AA. Bibliography#JLS 05]] |
]]></ac:plain-text-body></ac:structured-macro> |