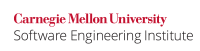
According to the Java Language Specification [JLS 2005], Section 11.2, "Compile-Time Checking of Exceptions,"
The unchecked exceptions classes are the class
RuntimeException
and its subclasses, and the classError
and its subclasses. All other exception classes are checked exception classes.
Unchecked exception classes such as Error
and its subclasses are not subject to compile-time checking because it is tedious to account for all exceptional conditions and recovery is generally difficult. However, recovery is usually possible, or a graceful exit that logs the error is at least feasible.
Noncompliant Code Example
This noncompliant code example generates a StackOverflowError
as a result of infinite recursion. It exhausts the available stack space and may result in denial of service.
public class StackOverflow { public static void main(String[] args) { infiniteRun(); // ... } private static void infiniteRun() { infiniteRun(); } }
Compliant Solution
This compliant solution shows a try-catch
block that can be used to capture java.lang.Error
or java.lang.Throwable
. A log entry can be made at this point and followed by attempts to free key system resources in the finally
block.
public class StackOverflow { public static void main(String[] args) { try { infiniteRun(); } catch(Throwable t) { // Forward to handler } finally { // Free cache, release resources } // ... } private static void infiniteRun() { infiniteRun(); } }
Note that this solution catches Throwable
in an attempt to handle the error and is an exception to guideline ERR08-J. Do not catch NullPointerException or any of its ancestors .
Risk Assessment
Allowing a system error to propagate out of a Java program may result in a denial-of-service attack.
Guideline | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
ERR53-JG | low | unlikely | medium | P2 | L3 |
Automated Detection
Automated detection is not feasible.
Related Guidelines
C++ Secure Coding Standard: ERR30-CPP. Try to recover gracefully from unexpected errors
Bibliography
[JLS 2005] Section 11.2, Compile-Time Checking of Exceptions
[Kalinovsky 2004] Chapter 16, Intercepting Control Flow: Intercepting System Errors