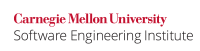
The enhanced for
statement designed for iteration through Collections and arrays .
The JLS provides the following example of the enhanced for
statement in §14.14.2, "The Enhanced for
Statement" [JLS 2014]:
The enhanced for statement is equivalent to a basic for statement of the form:
for (I #i = Expression.iterator(); #i.hasNext(); ) {
Unknown macro: {VariableModifier}TargetType Identifier =
(TargetType) #i.next();
Statement
}#i is an automatically generated identifier that is distinct from any other identifiers (automatically generated or otherwise) that are in scope...at the point where the enhanced for statement occurs.
Unlike the basic for
statement, assignments to the loop variable fail to affect the loop's iteration order over the underlying set of objects. Consequently, an assignment to the loop variable is equivalent to modifying a variable local to the loop body whose initial value is the object referenced by the loop iterator. This modification is not necessarily erroneous, but can obscure the loop functionality or indicate a misunderstanding of the underlying implementation of the enhanced for
statement.
Declare all enhanced for
statement loop variables final. The final declaration causes Java compilers to flag and reject any assignments made to the loop variable.
Noncompliant Code Example
This noncompliant code example attempts to process a collection of objects using an enhanced for
loop. It further intends to skip processing one item in the collection.
Collection<ProcessObj> processThese = // ... for (ProcessObj processMe: processThese) { if (someCondition) { // found the item to skip someCondition = false; processMe = processMe.getNext(); // attempt to skip to next item } processMe.doTheProcessing(); // process the object }
The attempt to skip to the next item appears to succeed because the assignment is successful and the value of processMe
is updated. Unlike a basic for
loop, however, the assignment leaves the overall iteration order of the loop unchanged. Consequently, the object following the skipped object is processed twice.
Note that if processMe
were declared final, a compiler error would result at the attempted assignment.
Compliant Solution
This compliant solution correctly processes each object in the collection no more than once.
Collection<ProcessObj> processThese = // ... for (final ProcessObj processMe: processThese) { if (someCondition) { // found the item to skip someCondition = false; continue; // skip by continuing to next iteration } processMe.doTheProcessing(); // process the object }
Risk Assessment
Assignments to the loop variable of an enhanced for
loop (for-each idiom) fail to affect the overall iteration order, lead to programmer confusion, and can leave data in a fragile or inconsistent state.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
DCL02-J | low | unlikely | low | P3 | L3 |
Automated Detection
This rule is easily enforced with static analysis.
Bibliography
01. Declarations and Initialization (DCL) 02. Expressions (EXP)