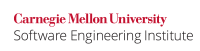
Exceptions should only be used to denote exceptional conditions. They should not be used for ordinary control flow purposes. Failure to follow this advice can result in abnormal control flow and performance degradation issues.
Noncompliant Code Example
This noncompliant code example attempts to concatenate the string elements of array values
and store the result as the first element.
String values[] = new String[3]; values[0] = "value1"; values[1] = "value2"; values[2] = "value3"; int i; values[1] = null; // gets null value try { i = 0; while(true) { values[0] = values[0].concat(values[i + 1]); // Concatenate and store in values[0] i++; } } catch (ArrayIndexOutOfBoundsException e) { i = 0; // Attempts to initialize i to 0 } catch (NullPointerException npe) { // Ignores }
It uses an ArrayIndexOutOfBoundsException
to detect the end of the array and reinitialize the value of variable i
to 0 in the catch
block. However, if some element of the array is null
, a NullPointerException
results. This exception is caught and ignored, a violation of EXC15-J. Do not catch NullPointerException. Consequently, the variable i
is not reinitialized to 0 in the catch
block.
The real purpose of exception handling is to detect and recover from exceptional conditions and not to willfully transfer control flow. Besides, performance wise, the exception-based idiom is far slower than the standard code block. It also prevents certain optimizations that the JVM would otherwise perform.
Compliant Solution
This compliant solution uses a standard for
loop to concatenate the strings.
String values[] = new String[3]; values[0] = "value1"; values[1] = "value2"; values[2] = "value3"; int i; for (i = 1; i < values.length; i++) { values[0] = values[0].concat(values[i]); } i = 0; // Initialize i to 0 after operation
Risk Assessment
Using exceptions for anything but detecting and handling exceptional conditions can result in performance degradation and poor design.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXC02- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Bloch 2001]] Item 39: "Use exceptions only for exceptional conditions"
[[JLS 2005]]
EXC01-J. Use a class dedicated to reporting exceptions 17. Exceptional Behavior (EXC) EXC03-J. Use a logging API to log critical security exceptions