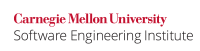
The varargs feature was introduced in the JDK v1.5.0. Its utility lies in allowing a method to accept a variable number of arguments.
According to the Java SE 6 documentation [[Sun 2006]] varargs
As an API designer, you should use [varargs methods] sparingly, only when the benefit is truly compelling. Generally speaking, you should not overload a varargs method, or it will be difficult for programmers to figure out which overloading gets called.
Noncompliant Code Example
Overloading varargs methods can create confusion as shown in this noncompliant code example. The programmer's intent is to invoke the variable argument (varargs) doSomething()
method, but instead its overloaded, more specific form takes precedence.
class OverloadedVarargs { private static void doSomething(boolean... bool) { System.out.print("Number of arguments: " + bool.length + ", Contents: "); for (boolean b : bool) System.out.print("[" + b + "]"); } private static void doSomething(boolean bool1, boolean bool2) { System.out.println("Overloaded method invoked"); } public static void main(String[] args) { doSomething(true, false); } }
Compliant Solution
Avoid overloading varargs methods. Use distinct method names so that the intended method gets invoked as prescribed by this compliant solution.
class NotOverloadedVarargs { private static void doSomething1(boolean... bool) { System.out.print("Number of arguments: " + bool.length + ", Contents: "); for (boolean b : bool) System.out.print("[" + b + "]"); } private static void doSomething2(boolean bool1, boolean bool2) { System.out.println("Overloaded method invoked"); } public static void main(String[] args) { doSomething1(true, false); } }
Exceptions
DCL08-EX1: Sometimes, it is desirable to violate the "do not overload varargs methods" advice for performance reasons (avoiding the cost of creation of an array instance and its initialization on every invocation of a varargs method). [[Bloch 2008]]
public void foo() { } public void foo(int a1) { } public void foo(int a1, int a2, int... rest) { }
The idiom shown above avoids the pitfalls of incorrect method selection by using non-ambiguous method signatures and can be discreetly used where required.
Risk Assessment
Unmindful use of the varargs feature may create ambiguity and diminish code readability.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL08-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Sun 2006]] varargs
[[Bloch 2008]] Item 42: "Use varargs judiciously"
[[Steinberg 2005]] "Using the Varargs Language Feature"
DCL07-J. Beware of integer literals beginning with '0' 03. Declarations and Initialization (DCL) DCL09-J. Enforce compile-time type checking of variable argument types