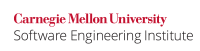
Exceptions should only be used to denote exceptional conditions. They should not be used for ordinary control flow purposes.
Noncompliant Code Example
This noncompliant code example attempts to concatenate a few string elements of array c
and store the result as the first element. It uses an ArrayIndexOutOfBoundsException
to detect the end of the array and initialize the value of variable i
to 0 in the catch
block. However, if some element of the array is null
, a NullPointerException
results. This exception is caught and ignored, a violation of EXC15-J. Do not catch NullPointerException. Consequently, the variable i
is not initialized to 0 as expected.
String c[] = new String[3]; c[0] = "value1"; c[1] = "value2"; c[2] = "value3"; int i; c[1] = null; // gets null value try { i = 0; while(true) { c[0] = c[0].concat(c[i + 1]); // Concatenate and store in c[0] i++; } } catch (ArrayIndexOutOfBoundsException e) { i = 0; // Attempts to initialize i to 0 } catch (NullPointerException npe) { // Ignores }
The real purpose of exception handling is to detect and recover from exceptional conditions and not to willfully transfer control flow. Besides, performance wise, the exception-based idiom is far slower than the standard code block. It also prevents certain optimizations that JVM would otherwise perform.
Compliant Solution
This compliant solution uses a standard for
loop to concatenate the strings.
String c[] = new String[3]; c[0] = "value1"; c[1] = "value2"; c[2] = "value3"; int i; for (i = 1; i < c.length; i++) { c[0] = c[0].concat(c[i]); } i = 0; // Initialize i to 0 after operation
Risk Assessment
The use of exceptions for anything but detecting and handling exceptional conditions can result in performance degradation and poor design.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXC02- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Bloch 01]] Item 39: "Use exceptions only for exceptional conditions"
[[JLS 05]]
CON21-J. Facilitate thread reuse by using Thread Pools 11. Concurrency (CON) CON08-J. Do not call overridable methods from synchronized regions