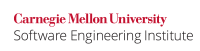
Mutable classes are those which when instantiated, return a reference such that the contents of the instance can be altered. It is important to provide means for creating copies of mutable classes to allow passing their respective class objects to untrusted code. The copying ensures that untrusted code cannot manipulate the object's state. The copying mechanism can be advertised by overriding java.lang.Object
's clone()
method. However, providing a clone()
method by itself, does not obviate the need to create defensive copies when receiving input from untrusted code and returning values to the same. Only a trusted caller can be expected to responsibly call the overriding clone()
method before passing instances to untrusted code.
Noncompliant Code Example
In this noncompliant code example, MutableClass
declares a mutable Date
object. If the trusted caller passes the Date
instance to untrusted code, and the latter changes the instance of the Date
object (like incrementing the month or changing the timezone), the class implementation no longer remains consistent with its old state. This problem can also arise in the presence of multiple threads.
public final class MutableClass { private Date d; public MutableClass(Date d) { this.d = d; } public Date getDate() { return d; } }
Compliant Solution
Always provide mechanisms to create copies of the instances of a mutable class. This compliant solution implements the Cloneable
interface and overrides the clone
method to create a deep copy of the object. If it is possible that the accessor methods can be called from untrusted code, it is also advisable to copy all the mutable objects that are passed in before they are stored in the class (FIO31-J. Defensively copy mutable inputs and mutable internal components) or returned from any methods (OBJ37-J. Defensively copy private mutable class members before returning their references). This is because untrusted code cannot be expected to call the object's clone()
method to operate on the copy. This compliant solution provides a clone()
method to trusted code and also guarantees that the state of the object cannot be compromised when the accessor method is called directly from untrusted code.
public final class CloneClass implements Cloneable { private Date d; public CloneClass(Date d) { this.d = new Date(d.getTime()); //copy-in } public Object clone() throws CloneNotSupportedException { final CloneClass cloned = (CloneClass)super.clone(); cloned.d = (Date)d.clone(); //copy mutable Date object manually return cloned; } public Date getDate() { return (Date)d.clone(); //copy and return } }
At times, a mutable class's member class field is declared final
with no accessible copy methods. Callers can use the clone()
method to obtain an instance of the mutable class. This clone()
method must create a new instance of the final
member class and copy the original state to it. The new instance is necessary because no accessible copy method may be available in the member class. If the member class evolves in the future, it is critical to include the new state in the manual copy. [[SCG 07]]
As far as possible, a mutable class that defines a clone()
method, must be declared final
. This ensures that untrusted code cannot subclass and override the clone()
method to supply a spurious instance. Also refer to FIO31-J. Defensively copy mutable inputs and mutable internal components.
Exceptions
EX1: Sensitive classes should not be made cloneable. (MSC37-J. Make sensitive classes noncloneable)
Risk Assessment
Creating a mutable class without a clone
method may result in the data of its instance becoming corrupted when the instance is passed to untrusted code.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ36- J |
low |
likely |
medium |
P6 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] method clone()
[[Security 06]]
[[SCG 07]] Guideline 2-2 Support copy functionality for a mutable class
[[Bloch 08]] Item 39: Make defensive copies when needed
[[MITRE 09]] CWE ID 374 "Mutable Objects Passed by Reference", CWE ID 375
"Passing Mutable Objects to an Untrusted Method"
OBJ35-J. Use checked collections against external code 07. Object Orientation (OBJ) OBJ37-J. Defensively copy private mutable class members before returning their references