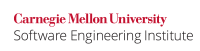
Holding locks while performing time consuming or blocking operations can severely degrade system performance and result in starvation. Furthermore, deadlock may result if too many interdependent threads block. Blocking operations include network, file and console I/O (for instance, invoking a method such as Console.readLine()
) and object serialization. Deferring a thread indefinitely also constitutes a blocking operation.
If the Java Virtual Machine (JVM) interacts with a file system that operates over an unreliable network, file I/O might incur a performance penalty. In such cases, avoid file I/O over the network while holding a lock. File operations such as logging that may block waiting for the output stream lock or for I/O to complete may be performed in a dedicated thread to speed up task processing. Logging requests can be added to a queue given that the put()
operation incurs little overhead as compared to file I/O [[Goetz 06, pg 244]].
Noncompliant Code Example (intrinsic lock)
This noncompliant code example defines a utility method that accepts a time
parameter.
private synchronized void doSomething(long time) throws InterruptedException { // ... Thread.sleep(time); }
Because the method is synchronized
, if the thread is suspended, other threads are unable to use the synchronized
methods of the class. In other words, the current object's monitor is not released. This is because the Thread.sleep()
method does not have any synchronization semantics, as detailed in CON16-J. Do not expect sleep(), yield() and getState() methods to have any synchronization semantics.
Compliant Solution (intrinsic lock)
This compliant solution defines the doSomething()
method with a timeout
parameter instead of the time
value. The use of the Object.wait()
method instead of Thread.sleep()
allows setting a time out for a period during which a notify signal may awaken the thread.
private synchronized void doSomething(long timeout) throws InterruptedException { while (<condition does not hold>) { wait(timeout); // Immediately releases lock on current monitor } }
The current object's monitor is immediately released upon entering the wait state. After the time out period has elapsed, the thread attempts to reacquire the current object's monitor and resumes execution when it succeeds.
According to the Java API [[API 06]], class Object
documentation:
Note that the
wait
method, as it places the current thread into the wait set for this object, unlocks only this object; any other objects on which the current thread may be synchronized remain locked while the thread waits. This method should only be called by a thread that is the owner of this object's monitor.
Consequently, ensure that a thread that holds locks on other objects releases them appropriately, before entering the wait state. Also, refer to the related guidelines CON18-J. Always invoke wait() and await() methods inside a loop and CON19-J. Notify all waiting threads instead of a single thread.
Noncompliant Code Example
This noncompliant code example shows the method sendPage()
that sends a Page
object from a server to a client. The method is synchronized so that the array pageBuff
is accessed safely when multiple threads request concurrent access.
// Class Page is defined separately. It stores and returns the Page name via getName() public final boolean SUCCESS = true; public final boolean FAILURE = false; Page[] pageBuff = new Page[MAX_PAGE_SIZE]; public synchronized boolean sendPage(Socket socket, String pageName) throws IOException { // Get the output stream to write the Page to ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream()); // Find the Page requested by the client (this operation requires synchronization) Page targetPage = null; for(Page p : pageBuff) { if(p.getName().compareTo(pageName) == 0) { targetPage = p; } } // Requested Page does not exist if(targetPage == null) { return FAILURE; } // Send the Page to the client (does not require any synchronization) out.writeObject(targetPage); out.flush(); out.close(); return SUCCESS; }
Calling writeObject()
within the synchronized sendPage()
method can result in delays and deadlock-like conditions in high latency networks or when network connections are inherently lossy.
Compliant Solution
This compliant solution entails separating the actions into a sequence of steps:
- Perform actions on data structures requiring synchronization
- Create copies of the objects that are required to be sent
- Perform network calls in a separate method that does not require any synchronization
In this compliant solution, the synchronized method getPage()
is called from an unsynchronized method sendPage()
, to find the requested Page
in the pageBuff
array. After the Page
is retrieved, the method sendPage()
calls the unsynchronized method deliverPage()
to deliver the Page
to the client.
public boolean sendPage(Socket socket, String pageName) { // No synchronization Page targetPage = getPage(pageName); if(targetPage == null) return FAILURE; return deliverPage(socket, targetPage); } private synchronized Page getPage(String pageName) { // Requires synchronization Page targetPage = null; for(Page p : pageBuff) { if(p.getName().equals(pageName)) { targetPage = p; } } return targetPage; } public boolean deliverPage(Socket socket, Page page){ try{ // Get the output stream to write the Page to ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream()); // Send the Page to the client out.writeObject(page); } catch(IOException io){ // If recovery is not possible return FAILURE return FAILURE; } finally { out.flush(); out.close(); } return SUCCESS; }
Exceptions
EX1: Classes that are compliant with the guideline CON24-J. Ensure that threads and tasks performing blocking operations can be terminated in that they provide an appropriate termination mechanism to callers, are allowed to violate this guideline.
EX2: A method that requires multiple locks may hold several locks while waiting for the remaining locks to become available. This constitutes a valid exception, though care must be taken to avoid deadlock. See CON12-J. Avoid deadlock by requesting and releasing locks in the same order for more information.
Risk Assessment
If blocking or time consuming operations are performed within synchronized regions, temporary or permanent deadlock may result.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON20-J |
low |
probable |
high |
P2 |
L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Class Object
[[Grosso 01]] Chapter 10: Serialization
[[JLS 05]] Chapter 17, Threads and Locks
[[Rotem 08]] Falacies of Distributed Computing Explained
CON19-J. Notify all waiting threads instead of a single thread 11. Concurrency (CON) CON21-J. Facilitate thread reuse by using Thread Pools