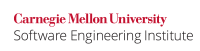
Mutable classes allow code external to the class to alter their instance or class fields. It is important to provide means for creating copies of mutable classes to allow passing their respective class objects to untrusted code. This functionality is useful when methods in other classes need to create copies of the particular class instance (see FIO00-J. Defensively copy mutable inputs and mutable internal components and OBJ11-J. Defensively copy private mutable class members before returning their references).
The copying mechanism can be advertised by overriding java.lang.Object
's clone()
method. Use of the clone()
method is only secure for final
classes. Nonfinal classes must either provide a copy constructor or a public static factory method that returns a copy of the instance.
On its own, providing copy functionality does not obviate the need of creating defensive copies of input received from untrusted code, and of output that is returned to the same. Only a trusted caller can be expected to responsibly use the copy functionality of the class before passing the object instance to untrusted code.
Noncompliant Code Example
In this noncompliant code example, MutableClass
uses a mutable field date
of type Date
. The instance member Date
is also mutable.
public final class MutableClass { private Date date; public MutableClass(Date d) { this.date = d; } public void setDate(Date d) { this.date = d; } public Date getDate() { return date; } }
If a trusted caller passes an instance of MutableClass
to untrusted code, and the latter changes the instance of the Date
object (like incrementing the month or changing the timezone), the state of the object no longer remains consistent with its old state. This problem can also arise in the presence of multiple threads.
Compliant Solution (nonfinal instance members)
This compliant solution implements the Cloneable
interface and overrides the Object.clone()
method to create a deep copy of the object.
public final class MutableClass implements Cloneable { private Date date; public MutableClass(Date d) { this.date = new Date(d.getTime()); //copy-in } public Date getDate() { return (Date)date.clone(); //copy and return } public Object clone() throws CloneNotSupportedException { final MutableClass cloned = (MutableClass)super.clone(); cloned.date = (Date)date.clone(); //copy mutable Date object manually return cloned; } }
Note that the clone()
method must manually clone the Date
object. This step is usually unnecessary when the object contains only primitive fields or fields that refer to immutable objects. When the fields contain data such as unique identifiers or object creation times, the new values of the fields must be calculated and assigned manually in the clone()
method [[Bloch 2008]].
A mutable class that defines a clone()
method must be declared final
. This ensures that untrusted code cannot subclass and override the clone()
method to supply a spurious instance. The clone()
method should copy all internal mutable state as necessary. In this compliant solution, the Date
object is copied.
Also, if untrusted code can call accessor methods and pass mutable arguments, it is advisable to copy the arguments before they are stored in any instance fields (FIO00-J. Defensively copy mutable inputs and mutable internal components). When retrieving internal mutable state, a copy of the members must be created before returning their references to untrusted code (OBJ11-J. Defensively copy private mutable class members before returning their references).
This would be unnecessary if untrusted code were to call object's clone()
method to operate on the copy, however, untrusted code has little incentive to do so. This compliant solution provides a clone()
method to trusted code and also guarantees that the state of the object cannot be compromised when the accessor methods are called directly from untrusted code.
Compliant Solution (instance members declared final)
Sometimes, a mutable class's instance fields are declared final
with no accessible copy methods. In this case, a clone()
method should be provided as shown in this compliant solution.
public final class MutableClass implements Cloneable { private final Date date; // final field public MutableClass(Date d) { this.date = new Date(d.getTime()); //copy-in } public Date getDate() { return (Date)date.clone(); //copy and return } public Object clone() throws CloneNotSupportedException { Date d = new Date(); d = (Date)date.clone(); MutableClass cloned = new MutableClass(d); return cloned; } }
Callers can use the clone()
method to obtain an instance of such a mutable class. The clone()
method must create a new instance of the final
member class and copy the original state to it. The new instance is necessary because no accessible copy method may be available in the member class. If the member class evolves in the future, it is critical to include the new state in the manual copy. Finally, the clone()
method must create and return a new instance of the enclosing class (MutableClass
) using the newly created member instance (d
). [[SCG 2007]]
Compliant Solution (copy constructor)
This compliant solution uses a copy constructor that initializes a MutableClass
instance when an argument of the same type (or subtype) is passed to it.
public final class MutableClass { // Copy Constructor private final Date date; public MutableClass(MutableClass mc) { this.date = new Date(mc.date.getTime()); } public MutableClass(Date d) { this.date = new Date(d.getTime()); // Copy-in } public Date getDate() { return (Date)date.clone(); // Copy and return } }
This approach is useful when the instance fields are declared final
. A copy is requested by invoking the copy constructor with an existing MutableClass
instance as argument.
Compliant Solution (public static factory method)
This compliant solution exports a public static factory method getInstance()
that creates and returns a copy of a given MutableClass
object instance.
class MutableClass { private final Date date; private MutableClass(Date d) { // Noninstantiable and nonsubclassable this.date = new Date(d.getTime()); // Copy-in } public Date getDate() { return (Date)date.clone(); // Copy and return } public static MutableClass getInstance(MutableClass mc) { return new MutableClass(mc.getDate()); } }
This approach is useful when the instance fields are declared final
.
Exceptions
EX1: Sensitive classes should not be made cloneable. (MSC05-J. Make sensitive classes noncloneable)
Risk Assessment
Creating a mutable class without without providing copy functionality may result in the data of its instance becoming corrupted when the instance is passed to untrusted code.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ10- J |
low |
likely |
medium |
P6 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 2006]] method clone()
[[Security 2006]]
[[SCG 2007]] Guideline 2-2 Support copy functionality for a mutable class
[[SCG 2009]] Guideline 2-3 Support copy functionality for a mutable class
[[Bloch 2008]] Item 39: Make defensive copies when needed and Item 11: Override clone judiciously
[[MITRE 2009]] CWE ID 374 "Mutable Objects Passed by Reference", CWE ID 375
"Passing Mutable Objects to an Untrusted Method"
OBJ09-J. Immutable classes must prohibit extension 08. Object Orientation (OBJ) OBJ11-J. Defensively copy private mutable class members before returning their references