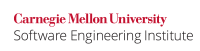
The final
keyword can be used to specify constant values (that is, values that cannot change during program execution). However, constants that can change over the lifetime of a program should not be declared public final. The Java Language Specification allows implementations to insert the values of public final fields inline in any compilation unit that reads the field. Consequently, if the declaring class is edited so that the new version gives a different value for the field, compilation units that read the public final field could still see the old value until they are recompiled.
A related error can arise when a programmer declares a static final
reference to a mutable object; see guideline "VOID OBJ02-J. Never conflate immutability of a reference with that of the referenced object" for additional information.
Noncompliant Code Example
In this noncompliant code example, class Foo
declares a field whose value represents the version of the software. The field is subsequently accessed by class Bar
from a separate compilation unit.
Foo.java
:
class Foo { public static final int VERSION = 1; // ... }
Bar.java
:
class Bar { public static void main(String[] args) { System.out.println("You are using version " + Foo.VERSION); } }
When compiled and run, the software correctly prints
You are using version 1
But if a developer changes the value of VERSION
to 2 by modifying Foo.java
and recompiles Foo.java
but fails to recompile Bar.java
, the software incorrectly prints:
You are using version 1
Although recompiling Bar.java
solves this problem, a better solution is available.
Compliant Solution
According to §13.4.9, "final
Fields and Constants" of the Java Language Specification [[JLS 2005]],
Other than for true mathematical constants, we recommend that source code make very sparing use of class variables that are declared
static
andfinal
. If the read-only nature offinal
is required, a better choice is to declare aprivate static
variable and a suitable accessor method to get its value.
Consequently, a compliant solution is:
Foo.java
:
class Foo { private static final int version = 1; public static final String getVersion() { return version; } // ... }
Bar.java
:
class Bar { public static void main(String[] args) { System.out.println("You are using version " + Foo.getVersion()); } }
In this solution, the private version value cannot be copied into the Bar
class when it is compiled, consequently preventing the bug. Note that most just-in-time (JIT) code generators can inline the getVersion()
method at runtime, so little or no performance penalty is incurred.
Exceptions
DCL04-EX1: According to §9.3, "Field (Constant) Declarations" of the Java Language Specification [[JLS 2005]],
"Every field declaration in the body of an interface is implicitly public
, static
, and final
. It is permitted to redundantly specify any or all of these modifiers for such fields."
DCL04-EX2: Constants declared using the enum
type are permitted to violate this guideline.
DCL04-EX3: Constants whose value never changes throughout the entire lifetime of the software may be declared as final
. For instance, the Java Language Specification recommends that mathematical constants be declared final
.
Risk Assessment
Failing to declare mathematical constants static
and final
can lead to thread-safety issues as well as to inconsistent behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL04-J |
low |
probable |
medium |
P2 |
L3 |
Automated Detection
Static checking of this guideline is not feasible in the general case.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Secure Coding Standard: DCL00-C. Const-qualify immutable objects
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="a44cf542-20a0-4b67-92a6-ce3cc59c90b0"><ac:plain-text-body><![CDATA[ |
[[JLS 2005 |
AA. References#JLS 05]] |
[§13.4.9, "final Fields and Constants" |
http://java.sun.com/docs/books/jls/third_edition/html/binaryComp.html#13.4.9] |
]]></ac:plain-text-body></ac:structured-macro> |
|
|||||
|
|||||
|
01. Declarations and Initialization (DCL) DCL05-J. Declare all enhanced for statement loop variables to be final