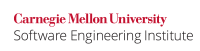
The conditional operator ?:
uses the boolean
value of one expression to decide which of the other two expressions should be evaluated [[JLS 05]].
The conditional operator is syntactically right-associative. For instance a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
The general form of a Java conditional expression is operand1 ? operand2 : operand3
.
- If the value of the first operand (
operand1
) istrue
, then the second operand expression (operand2
) is chosen - If the value of the first operand is
false
, then the third operand expression (operand3
) is chosen
The rules used by a Java compiler to determine the type of the result of a conditional expression are quite complicated and may result in unexpected type conversions. The rules used to determine the result type of a conditional expression are given in the following table, where the first matching rule, starting from the top, is used. In the table, *
refers to constant expressions of type int
(such as '0' or variables declared final
), Operand 2 refers to operand2
in the general form of a Java conditional given above, and Operand 3 refers to operand3
:
Operand 2 |
Operand 3 |
Resultant type |
---|---|---|
type T |
type T |
type T |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
other numeric |
other numeric |
promoted type of the 2nd and 3rd operands |
T1 = boxing conversion (S1) |
T2 = boxing conversion(S2) |
apply capture conversion to lub(T1,T2) |
Noncompliant Code Example
This noncompliant example prints A6565
instead of AAA
.
- The first print statement prints the value of
alpha
asA
, which is of thechar
type. The third operand '0', is a constant expression of typeint
whose value can be represented as achar
and hence does not cause any numeric promotion. - The second statement prints
65
, the integer equivalent ofA
. This is because of numeric promotion of the second operandalpha
to anint
which happens because the third operand, the constant expression '12345', is anint
that cannot be represented as achar
. - The third statement also prints
65
. This is because of numeric promotion of the third operandalpha
to anint
, which happens because the second operand, variablei
, is anint
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; System.out.print(true ? alpha : 0); System.out.print(true ? alpha : 12345); System.out.print(false ? i : alpha); } }
Compliant Solution
This compliant solution recommends the use of the same types for the second and third operands of the conditional expressions. The clearer semantics help avoid confusion.
public class Expr { public static void main(String[] args) { char alpha = 'A'; char i = 0; //declare as char System.out.print(true ? alpha : 0); // Cast alpha as an int to explicitly state that the type of the // conditional expression should be int. System.out.print(true ? ((int) alpha) : 12345); System.out.print(false ? i : alpha); } }
Note that while casting 12345
to type char
would ensure that both operands in the second nonconforming conditional expression have the same type, it would result in data loss when 12345
is converted to a char
. Therefore the conforming example casts alpha
to int
, the wider of the operand types.
Risk Assessment
If the types of the second and third operands in a conditional expression are not the same then the result of the conditional expression may be unexpected.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP00- J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[JLS 05]] Section 15.25, Conditional Operator ? :
[[Bloch 05]] Puzzle 8: Dos Equis
03. Expressions (EXP) 03. Expressions (EXP) EXP01-J. Ensure a null pointer is not dereferenced