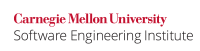
In the presence of a security manager, it is hard for malicious code to exploit Java's security model. For example, instantiating sensitive classes such as a ClassLoader
is prohibited in the context of a web browser. It is critical to ensure that untrusted code does not indirectly use the privileges of legit code. Failure to do so can leave the code vulnerable to privilege escalation attacks. This is because, classes loaded by the same class loader exist in the same namespace. Consider for example, an untrusted method calling a class method which loads classes using its own trusted class loader. This is a problem as untrusted code's class loader may not have the permission to load the particular class. Also, if the trusted code accepts tainted inputs, it is susceptible to exploits resulting from malicious classes getting loaded.
The APIs tabulated here perform tasks using the immediate caller's, trusted class loader.
APIs |
---|
java.lang.Class.forName |
java.lang.Package.getPackage(s) |
java.lang.Runtime.load |
java.lang.Runtime.loadLibrary |
java.lang.System.load |
java.lang.System.loadLibrary |
java.sql.DriverManager.getConnection |
java.sql.DriverManager.getDriver(s) |
java.sql.DriverManager.deregisterDriver |
java.util.ResourceBundle.getBundle |
Noncompliant Code Example
The untrustedCode
method of class Untrusted
invokes loadLib
method of class NativeCode
in this noncompliant example. This is dangerous as the library gets loaded on behalf of the untrusted code. Accepting tainted inputs from untrusted code can further exacerbate this issue. In essence, the untrusted code's class loader may be able to load the intended library even if it does not have sufficient permissions.
class NativeCode { public native void loadLib(); static { try { System.loadLibrary("/com/foo/MyLib.so"); }catch(UnsatisfiedLinkError e) { e.getMessage(); } } } class Untrusted { public static void untrustedCode() { new NativeCode().loadLib(); } }
Sometimes, a call to System.loadLibrary
is embedded in a doPrivileged
block, as shown below. An unprivileged caller can maliciously invoke this piece of code.
AccessController.doPrivileged(new PrivilegedAction() { public Object run() { System.loadLibrary("awt"); return null; } });
Noncompliant Code Example
The single argument Class.forname
method is another example of an API that uses its immediate caller's class loader to load a desired class. Untrusted code can indirectly abuse this API.
Class c = Class.forName(className);
Compliant Solution
Ensure that untrusted code cannot invoke the affected APIs directly or indirectly (that is, via a call to an invoking method). Do not operate on tainted inputs and make sure that internal objects are not returned to untrusted code.
Risk Assessment
Allowing untrusted code to load libraries using the immediate caller's class loader may seriously compromise the security of a java application.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC03-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[SCG 07]] Guideline 6-3 Safely invoke standard APIs that perform tasks using the immediate caller's class loader instance
SEC02-J. Do not expose standard APIs that may bypass Security Manager checks to untrusted code 00. Security (SEC) SEC04-J. Beware of standard APIs that perform access checks against the immediate caller