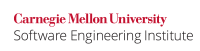
Non-final classes containing methods that perform security checks can be compromised if a malicious subclass overrides the methods and omits the checks. For this reason, these methods must be declared private or final to prevent them from being extended.
Noncompliant Code Example
This noncompliant code example allows a subclass to override the readSensitiveFile()
method and omit the required security check.
public void readSensitiveFile() { try { SecurityManager sm = System.getSecurityManager(); if (sm != null) { // Check if file can be read sm.checkRead("/temp/tempFile"); } // Access the file } catch (SecurityException se) { // Log exception } }
Compliant Solution
This compliant solution prohibits inheritance of the readSensitiveFile()
method by declaring it as final.
public final void readSensitiveFile() { try { SecurityManager sm = System.getSecurityManager(); if (sm != null) { // check if file can be read sm.checkRead("/temp/tempFile"); } // Access the file } catch (SecurityException se) { // Log exception } }
Compliant Solution
This compliant solution prohibits inheritance of the readSensitiveFile()
method by declaring it private.
private void readSensitiveFile() { try { SecurityManager sm = System.getSecurityManager(); if (sm != null) { // check if file can be read sm.checkRead("/temp/tempFile"); } // Access the file } catch (SecurityException se) { // Log exception } }
Exceptions
MET03-EX0: Classes that are declared final
are exempt from this guideline as they imply that the contained methods cannot be overridden.
Risk Assessment
Failing to declare a non-final class's method private or final can allow a subclass to circumvent the security checks performed in the methods.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET03-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Ware 2008]]
MET02-J. Validate method parameters 05. Methods (MET) MET04-J. Ensure that constructors do not call overridable methods