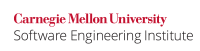
Returning references to internal mutable members of a class can seriously compromise the security of an application because of the resulting sub par encapsulation properties and susceptibility to corruption of the class data. A caller can modify the private
data if instead of defensive copies of mutable class members, direct references to them are returned.
Returning references that refer to private
data to untrusted code can be more pernicious than returning the references to trusted code. If a class defines a clone()
method that trusted code can use to pass defensive copies of the instance to untrusted code (OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely), the implementing class may violate this guideline. However, the burden is now transferred to the trusted code as it is expected to reliably call the clone()
method before operating on the instance or passing it to untrusted code.
Note that the performance costs of violating this guideline (or calling clone()
) might be significantly more than using an accessor method that returns a copy of a single mutable member. This is because a well designed clone()
method returns a copy of the complete object, including all mutable components) as opposed to that of a single member, which makes it relatively slower.
Pugh [[Pugh 09]] cites a vulnerability discovered by the Findbugs static analysis tool in the early betas of jdk 1.7. The class sun.security.x509.InvalidityDateExtension
returned a Date
instance through a public
accessor, without creating defensive copies.
Noncompliant Code Example
This noncompliant code example defines a class that contains a private
Hashtable
instance field. Here, the hash table itself is designed to hold immutable data of sensitive nature (SSN numbers). A getter method getValues()
gives the caller access to the hash table by returning a reference to it. When invoked by untrusted code, entries may be maliciously added, removed or replaced.
class ReturnRef { // Internal state, may contain sensitive data Hashtable<Integer,String> ht = new Hashtable<Integer,String>(); private ReturnRef() { ht.put(1, "123-45-6666"); } public Hashtable<Integer,String> getValues(){ return ht; } public static void main(String[] args) { ReturnRef rr = new ReturnRef(); Hashtable<Integer, String> ht1 = rr.getValues(); // Prints sensitive data 123-45-6666 ht1.remove(1); // Untrusted caller can remove entries Hashtable<Integer, String> ht2 = rr.getValues(); // Now prints null, original entry is removed } }
Compliant Solution
Do not provide a getter method like getValues()
that exposes private
internal object state. This applies largely to members containing mutable as well as immutable data. Deep copies of mutable data are required to be returned whereas it suffices to return shallow copies of mutable fields containing immutable data.
This compliant solution creates and returns a shallow copy of the hash table containing immutable SSN numbers. As a result, any attempts to modify the original hash table are ineffective.
private Hashtable<Integer,String> getValues(){ return (Hashtable<Integer,String>)ht.clone(); // shallow copy } public static void main(String[] args) { ReturnRef rr = new ReturnRef(); Hashtable<Integer,String> ht1 = rr.getValues(); // prints non sensitive data ht1.remove(1); // untrusted caller can remove entries only from the copy Hashtable<Integer,String> ht2 = rr.getValues(); // prints non sensitive data }
If the hash table contained references to mutable data such as a series of Date
objects, every one of those objects must be copied by using a copy constructor or method. For further details, refer to FIO31-J. Defensively copy mutable inputs and mutable internal components and OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely. Note that the keys of a hash table need not be deep copied; shallow copying of the references suffices because a hash table's contract dictates that it cannot hold duplicate keys.
Noncompliant Code Example
This noncompliant code example shows a getDate()
accessor method that returns the sole instance of the private Date
object. An untrusted caller will be able to manipulate this instance as it exposes internal mutable components beyond the trust boundaries of the class.
class MutableClass { private Date d; public MutableClass() { d = new Date(); } protected Date getDate() { return d; } }
Compliant Solution
Do not carry out defensive copying using the clone()
method in constructors, when the (non-system) class can be subclassed by untrusted code. This will limit the malicious code from returning a crafted object when the object's clone()
method is invoked.
Despite this advice, this compliant solution recommends returning a clone of the Date
object. While this should not be done in constructors, it is permissible to use this technique in accessors. This is because there is no danger of a malicious subclass extending the internal mutable Date
object (Date
is a system class and consequently safe).
protected Date getDate() { return (Date)d.clone(); }
Arrays of primitive types are not exempt from this guideline. As arrays are objects in Java, if a reference to an array is returned, the caller may freely modify the contents of the original array. Shallow copies of arrays of primitive types are safe to return.
If the class has a public
setter method, this guideline still applies. Note that a setter method may perform input validation and sanitization before setting the internal fields. On the other hand, returning references to internal objects may require the caller to incorporate none of these defensive measures.
Exceptions
EX1: According to Sun's Secure Coding Guidelines document [[SCG 07]]:
if a class merely serves as a container for mutable inputs or outputs (the class does not directly operate on them), it may not be necessary to create defensive copies. For example, arrays and the standard collection classes do not create copies of caller-provided values. If a copy is desired so updates to a value do not affect the corresponding value in the collection, the caller must create the copy before inserting it into the collection, or after receiving it from the collection.
EX2: If the performance of the clone()
method is within reasonable bounds and the class clearly documents its use, this guideline may be violated. (OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely)
EX3: If the caller exposes an unmodifiable view of the object, it may not be required to defensively program the class to return copies of internal members. This decision should be made early in the design of the API. Note that if some other code wants to use this class in the future, it must also expose unmodifiable views. (SEC14-J. Provide sensitive mutable classes with unmodifiable wrappers)
Risk Assessment
Returning references to internal object state (mutable or immutable) can render an application susceptible to information leaks and corrupt the object's state and violate any class invariants. Control flow may also be affected in some cases.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ37- J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as OBJ35-CPP. Do not return references to private data.
References
[[API 06]] method clone()
[[Security 06]]
[[Bloch 08]] Item 39: Make defensive copies when needed
[[SCG 07]] Guideline 2-1 Create a copy of mutable inputs and outputs
[[Haggar 00]] Practical Java Praxis 64: Use clone for Immutable Objects When Passing or Receiving Object References to Mutable Objects
[[Goetz 06]] 3.2. Publication and Escape: Allowing Internal Mutable State to Escape
[[Gong 03]] 9.4 Private Object State and Object Immutability
[[MITRE 09]] CWE ID 375 "Passing Mutable Objects to an Untrusted Method"
OBJ36-J. Provide mutable classes with a clone method to allow passing instances to untrusted code safely 08. Object Orientation (OBJ) OBJ38-J. Immutable classes must prohibit extension