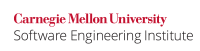
Code is usually signed when it requires more than the default set of permissions to perform some tasks. Although it is usually a bad idea to sign code, some actions necessitate this step. For example, if the application needs to establish an http
connection with an external host to download plugins or extensions, a vendor may provide signed code instead of having the user deal with complex security policies. Because executing signed code can be extremely dangerous, verifying authenticity of origin is of utmost importance.
Java based technologies typically use the Java Archive (JAR) feature for packaging files to facilitate platform independent deployment. Be it desktop applications, Enterprise Java Beans (EJB), MIDlets (J2ME) or Weblogic Server J2EE applications, JAR files are the preferred means of distribution. The point and click installation provided by Java Web Start also relies on the JAR file format for packaging. Vendors sign their JAR files when required, however, this should not be interpreted to be the case that the code cannot be misused.
According to the Java Tutorials [[Tutorials 08]]:
If you are creating applet code that you will sign, it needs to be placed in a JAR file. The same is true if you are creating application code that may be similarly restricted by running it with a security manager. The reason you need the JAR file is that when a policy file specifies that code signed by a particular entity is permitted one or more operations, such as specific file reads or writes, the code is expected to come from a signed JAR file. (The term "signed code" is an abbreviated way of saying "code in a class file that appears in a JAR file that was signed.")
Depending on how the client code works, signatures may or may not be automatically checked programatically. For example, any instances of URLClassLoader
and its subclasses and java.util.jar
automatically verify a signature whenever the JAR file is signed. If however, the developer implements a custom classloader that goes on to subclass java.lang.ClassLoader
, this step is not performed automatically. Moreover, in the URLClassLoader
case, the automatic verification just involves an integrity check and does not authenticate the loaded class. This is because the check uses a public key that is contained within the JAR. The legit JAR file may be replaced with a malicious JAR file containing a different public key and digest values.
Noncompliant Code Example
This noncompliant code example demonstrates the JarRunner
application that can be used to dynamically execute a particular class residing within a JAR file (abridged version of the class in [Tutorials 08]). It creates a JarClassLoader
that loads an application update, plugin or patch over an untrusted network such as the Internet. The URL to fetch the code is specified as the first argument (for example, http://somewebsite.com/software-updates.jar) and any other arguments specify the arguments that are to be passed to the class to be loaded. Reflection is used to invoke the
main
method of the loaded class. Unfortunately, by default, JarClassLoader
verifies the signature using the public key contained within the JAR file.
public class JarRunner { public static void main(String[] args) throws IOException, ClassNotFoundException,NoSuchMethodException, InvocationTargetException { URL url = new URL(args[0]); // Create the class loader for the application jar file JarClassLoader cl = new JarClassLoader(url); // Get the application's main class name String name = cl.getMainClassName(); // Get arguments for the application String[] newArgs = new String[args.length - 1]; System.arraycopy(args, 1, newArgs, 0, newArgs.length); // Invoke application's main class cl.invokeClass(name, newArgs); } } class JarClassLoader extends URLClassLoader { private URL url; public JarClassLoader(URL url) { super(new URL[] { url }); this.url = url; } public String getMainClassName() throws IOException { URL u = new URL("jar", "", url + "!/"); JarURLConnection uc = (JarURLConnection) u.openConnection(); Attributes attr = uc.getMainAttributes(); return attr != null ? attr.getValue(Attributes.Name.MAIN_CLASS) : null; } public void invokeClass(String name, String[] args) throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException { Class c = loadClass(name); Method m = c.getMethod("main", new Class[] { args.getClass() }); m.setAccessible(true); int mods = m.getModifiers(); if (m.getReturnType() != void.class || !Modifier.isStatic(mods) || !Modifier.isPublic(mods)) { throw new NoSuchMethodException("main"); } try { m.invoke(null, new Object[] { args }); } catch (IllegalAccessException e) { System.out.println("Access denied"); } } }
Compliant Solution
If the program expects the user to manually install the new JAR file, the user can explicitly check the signature from the command line. Any malicious tampering results in a SecurityException
when the jarsigner
tool is invoked with the -verify
option.
jarsigner -verify signed-updates-jar-file.jar
When the local system cannot reliably verify the signature, an explicit signature verification check must be built within the invoking program. This can be achieved by obtaining the chain of certificates from the CodeSource
of the class being loaded and checking if any one of the certificates belongs to the trusted signer whose certificate has been securely obtained beforehand and stored in a local keystore. The invokeClass
method can be modified to do this as shown in this compliant solution.
public void invokeClass(String name, String[] args) throws ClassNotFoundException, NoSuchMethodException, InvocationTargetException, GeneralSecurityException, IOException { Class c = loadClass(name); Certificate[] certs = c.getProtectionDomain().getCodeSource().getCertificates(); if(certs == null) { System.out.println("No signature!"); return; // return, do not execute if unsigned } KeyStore ks = KeyStore.getInstance("JKS"); ks.load(new FileInputStream(System.getProperty("user.home"+ File.separator + "keystore.jks")), "loadkeystorepassword".toCharArray()); Certificate pubCert = ks.getCertificate("user"); // user is the alias certs[0].verify(pubCert.getPublicKey()); // check with the trusted public key, else throws exception }
(Note that invokeClass
now has two more exceptions in its throws
clause, so the catch
block in the main
method must be altered accordingly.)
It is not always the case that arbitrary code gets executed. By default, the URLClassLoader
and all its subclasses are only given enough permissions to interact with the URL
that was specified when the URLClassLoader
object was created. This means that the program can only interact with the specified host. However, this does not mitigate the risk completely as the loaded file may have been granted appropriate privileges to perform other sensitive operations such as updating an existing local JAR file.
Risk Assessment
Not verifying the digital signature either manually or programmatically can lead to the execution of malicious code.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC04- J |
high |
probable |
medium |
P12 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[Gong 03]] 12.8.3 jarsigner
[[Eclipse 08]] JAR Signing and Signed bundles and protecting against malicious code
[[Halloway 01]]
[[Flanagan 05]] Chapter 24. The java.util.jar Package
[[Oaks 01]] Chapter 12: Digital Signatures, Signed Classes
[[Tutorials 08]] The JarRunner Class, Lesson: API and Tools Use for Secure Code and File Exchanges
and Verifying Signed JAR Files
[[JarSpec 08]] Signature Validation
[[Bea 08]]
[[Muchow 01]]
[[MITRE 09]] CWE ID 300 "Channel Accessible by Non-Endpoint (aka 'Man-in-the-Middle')", CWE ID 319
"Cleartext Transmission of Sensitive Information", CWE ID 494
"Download of Code Without Integrity Check", CWE ID 347
"Improperly Verified Signature"
SEC03-J. Do not use APIs that perform access checks against the immediate caller 02. Platform Security (SEC) SEC01-J. Minimize accessibility of classes and their members