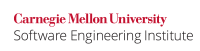
Storing sensitive information at client-side may result in its disclosure if an application is vulnerable to attacks that can compromise the information. For example, consider the use of a cookie for storing sensitive information such as user credentials. A cookie is set by a web server and is stored for a certain period of time on the client-side. All subsequent requests to the domain identified by the cookie are made to contain information that was saved in the cookie. If the web application is vulnerable to a cross-site scripting (XSS) vulnerability, an attacker may be able to read any unencrypted information contained in the cookie.
A partial list of sensitive information includes user names, passwords, password hashes, credit card numbers, social security numbers, and any other personally identifiable information about the user.
Noncompliant Code Example
In this noncompliant code example, the login servlet stores the user name and password in the cookie to identify the user for subsequent requests.
protected void doPost(HttpServletRequest request, HttpServletResponse response) { String username = request.getParameter("username"); char[] password = request.getParameter("password").toCharArray(); boolean rememberMe = Boolean.valueOf(request.getParameter("rememberme")); LoginService loginService = new LoginServiceImpl(); if (rememberMe) { if (request.getCookies()[0] != null && request.getCookies()[0].getValue() != null) { String[] value = request.getCookies()[0].getValue().split(";"); if (!loginService.isUserValid(value[0], value[1].toCharArray())) { // set error and return } else { // forward to welcome page } } else { boolean validated = loginService.isUserValid(username, password); if (validated) { Cookie loginCookie = new Cookie("rememberme", username + ";" + new String(password)); response.addCookie(loginCookie); // ... forward to welcome page } else { // set error and return } } } else { // no remember me functionality selected // proceed with regular authentication, if it fails set error and return } Arrays.fill(password, ' '); }
However, the attempt to implement the "remember me" functionality is insecure because sensitive information should not be stored at client-side without strong encryption.
Compliant Solution (Session)
- TODO -need to test the CS Dhruv Mohindra
This compliant solution implements the remember me functionality by storing the username and a secure random string in the cookie. It also maintains state in the session using HttpSession
.
protected void doPost(HttpServletRequest request, HttpServletResponse response) { // validate input (omitted) String username = request.getParameter("username"); char[] password = request.getParameter("password").toCharArray(); boolean rememberMe = Boolean.valueOf(request.getParameter("rememberme")); LoginService loginService = new LoginServiceImpl(); boolean validated = false; if (rememberMe) { if (request.getCookies()[0] != null && request.getCookies()[0].getValue() != null) { String[] value = request.getCookies()[0].getValue().split(";"); if(value.length != 2) { // set error and return } if (!loginService.mappingExists(value[0], value[1])) { // (username, random) validated = loginService.isUserValid(username, password); if (!validated) { // set error and return } } String newRandom = loginService.getRandomString(); // reset the random every time loginService.mapUserForRememberMe(username, newRandom); HttpSession session = request.getSession(); session.invalidate(); session = request.getSession(true); // Set session timeout to one hour session.setMaxInactiveInterval(60 * 60); // Store user attribute and a random attribute in session scope session.setAttribute("user", loginService.getUsername()); Cookie loginCookie = new Cookie("rememberme", username + ";" + newRandom); response.addCookie(loginCookie); // ... forward to welcome page } else { // ...authenticate using isUserValid() and if failed, set error } Arrays.fill(password, ' '); }
A mapping table is maintained at server side. The table contains username and secure random string pairs. When a user selects "remember me", the doPost
() method checks whether the supplied cookie contains a valid username and random string pair. If the mapping contains a matching pair, the user is authenticated and forwarded to the welcome page. If not, then an error is returned to the client. If the user selects "remember me" but the client does not supply a valid cookie, the user is made to authenticate using his credentials. If the authentication is successful, a new cookie is issued with "remember me" characteristics.
This solution also avoids session fixation attacks by invalidating the current session and creating a new session. It also reduces the window in which an attacker could perform a session hijacking attack by setting the session timeout to one.
Applicability
Violation of this rule places sensitive information within cookies, making the information vulnerable to packet sniffing or XSS attacks.
Related Guidelines
Bibliography