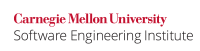
Synchronizing a class is sometimes unnecessary when a class is designed for single-threaded use. Such classes are required to document their thread-unsafe behavior. For instance, the documentation of class java.lang.StringBuilder
states:
This class is designed for use as a drop-in replacement for
StringBuffer
in places where the string buffer was being used by a single thread (as is generally the case). Where possible, it is recommended that this class be used in preference toStringBuffer
as it will be faster under most implementations.
Any multithreaded client must externally synchronize operations on a thread-unsafe object if the documentation of the corresponding class states that it is thread-unsafe.
However, classes that use mutable static
fields must always internally synchronize accesses to their fields. This is because there is no guarantee that all clients will synchronize externally when accessing the field. Because a static
field is shared by all clients, unrelated clients may violate the contract by not performing adequate synchronization.
Noncompliant Code Example
This noncompliant code example does not synchronize access to the static
field counter
.
class CountHits { private static int counter; public void incrementCounter() { counter++; } }
Consequently, there is no guarantee that all unrelated clients will externally synchronize accesses.
Compliant Solution
This compliant solution internally synchronizes the counter
field and does not depend on any external synchronization.
class CountHits { private static int counter; public synchronized void incrementCounter() { counter++; } }
Risk Assessment
Failing to internally synchronize classes containing accessible static members can result in unexpected results when a client fails to obey the external synchronization policy.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
CON32- J |
low |
probable |
medium |
P4 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]]
[[Bloch 08]] Item 67: "Avoid excessive synchronization"
VOID CON06-J. Do not defer a thread that is holding a lock 11. Concurrency (CON)