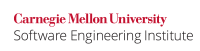
The java.io.InputStream
class is abstract
; programs thus require a wrapper such as BufferedInputStream
that provides a concrete implementation of the InputStream
. Java input classes often buffer the underlying input stream to facilitate fast, non-blocking I/O; Scanner
and BufferedInputStream
exemplify this approach.
Programs can create multiple wrappers on an InputStream
. Programs that encourage multiple wrappers around the same stream, however, behave significantly different depending on whether the InputStream
allows look-ahead or not. An adversary can exploit this difference in behavior by, for example, redirecting System.in
(from a file) or by using the System.setIn()
method to redirect System.in
. Note, however, that redirecting input from the console is a standard practice in UNIX based platforms; input redirection is less common on other platforms such as Windows, where console programs are largely considered outmoded. In general, any input stream that supports non-blocking buffered I/O is susceptible to this form of misuse.
Do not create multiple wrappers that buffer input from an InputStream
. Instead, create and use only one wrapper, either by passing it as an argument to the methods that need it or by declaring it as a class variable.
Noncompliant Code Example
This noncompliant code example creates multiple BufferedInputStream
wrappers on System.in
, even though there is only one declaration of a BufferedInputStream
. Each time getChar()
is called, it creates a new BufferedInputStream
. Because of the inherent channeling and buffering mechanism, data that has been read from the underlying stream cannot be replaced so that a second call can read the same data again. Consequently, data that remain in the buffer at the end of a particular execution of getChar()
are lost. Although this noncompliant code example uses a BufferedInputStream
, any buffered wrapper is unsafe; this condition is also exploitable if a Scanner
is used instead, for example.
public final class InputLibrary { public static char getChar() throws EOFException { BufferedInputStream in = new BufferedInputStream(System.in); // wrapper int input = in.read(); if (input == -1) { throw new EOFException(); } // Down casting is permitted because InputStream guarantees read() in range // 0..255 if it is not -1 return (char)input; } public static void main(String[] args) { try { // Either redirect input from the console or use // System.setIn(new FileInputStream("input.dat")); System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); // foward to handler } } }
Implementation Details
This program was compiled with the command javac InputLibrary.java
on a system with Java 1.6.0. When run from the command line with java InputLibrary
, the program successfully takes two characters as input and prints them out. However, when run with java InputLibrary < input
, where input
is a file that contains the exact same input, the program throws an EOFException
because the second call to getChar()
finds no characters to read upon encountering the end of the stream.
It may appear that the mark()
and reset()
methods of BufferedInputStream
could be used to replace the read bytes. However, these methods provide look-ahead by operating on the internal buffers of the BufferedInputStream
rather than operating directly on the underlying stream. Because the example code creates a new BufferedInputStream
on each call to getchar()
, the internal buffers of the previous BufferedInputStream
are lost.
Compliant Solution
Create and use only a single BufferedInputStream
on System.in
. This compliant solution ensures that all methods can access the BufferedInputStream
by declaring it as a class variable.
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); public static char getChar() throws EOFException { int input = in.read(); if (input == -1) { throw new EOFException(); } in.skip(1); // This statement is now necessary to go to the next line // The noncompliant code example deceptively worked without it return (char)input; } public static void main(String[] args) { try { System.out.print("Enter first initial: "); char first = getChar(); System.out.println("Your first initial is " + first); System.out.print("Enter last initial: "); char last = getChar(); System.out.println("Your last initial is " + last); } catch(EOFException e) { System.out.println("ERROR"); } } }
Implementation Details
This program was compiled with the command javac InputLibrary.java
on a system with Java 1.6.0. When run from the command line with java InputLibrary
, the program successfully takes two characters as input and prints them out. Also, when run with java InputLibrary < input
, where input
is a file that contains the exact same input, the program successfully takes two characters as input and print them out.
Compliant Solution
When a program intends to use the library InputLibrary
in conjunction with other code that requires user input and that consequently needs another buffered wrapper around System.in
, the program must use the same buffered wrapper as does the library, rather than creating and using its own additional buffered wrapper. The library InputLibrary
must return an instance of the buffered wrapper to support this functionality.
public final class InputLibrary { private static BufferedInputStream in = new BufferedInputStream(System.in); // Other methods static BufferedInputStream getBufferedWrapper() { return in; } } // Some code that requires user input from System.in class AppCode { private static BufferedInputStream in; AppCode() { in = InputLibrary.getBufferedWrapper(); } // Other methods }
Note that reading from a stream is not a thread-safe operation by default; consequently, this scheme may not work well in multi-threaded environments. Explicit synchronization is required in such cases.
Risk Assessment
Creating multiple buffered wrappers around an InputStream
can cause unexpected program behavior when the InputStream
is re-directed.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO05-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
Sound automated detection of this vulnerability is not feasible in the general case. Heuristic approaches may be useful.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[API 2006]] method read
[[API 2006]] class BufferedInputStream
FIO04-J. Canonicalize path names before validating 09. Input Output (FIO) FIO06-J. Ensure all resources are properly closed when they are no longer needed