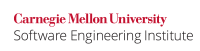
Cookies are an essential part of any web application and can be used for a number of different purposes such as user authentication. A cookie is a small piece of data that is set by a web server's response that will be stored for a certain period of time on the requesting user's computer. After a cookie has been set, all of the information within it will be sent in all subsequent requests to the cookie domain. Because of this, the information within a cookie is not secure and can be retrieved through a variety of cross-site scripting (XSS) attacks. As a result, it is important that the server does not set a cookie that contains excess or sensitive information about a user. This includes, but is not limited to, user names, passwords, password hashes, credit cards, and any personally identifiable information about the user.
Noncompliant Code Example
In this non compliant code example, we can see that the servlet stores the user name in the cookie to identify the user for authentication purposes.
private String login(HttpServletRequest request, HttpServletResponse response) {       List<String> errors = new ArrayList<String>();        request.setAttribute("errors", errors);              String username = request.getParameter("username");       String password = request.getParameter("password");       // Basic input validation       if(username.matches("[\\w]*")) errors.add("Incorrect user name format.");       if(password.matches("[\\w]*")) errors.add("Incorrect password format.");             if(errors.size() > 0) return "error.jsp";             UserBean dbUser = this.userDAO.lookup(username);       if(!dbUser.checkPassword(password)) {          errors.add("Passwords do not match.");          return "error.jsp";       }             Cookie userCookie = new Cookie("user", username); // Create a cookie that contains the username       response.addCookie(userCookie); // Send the cookie information to the client             return "welcome.jsp"; }
Note that the above non compliant code example stores the user name within the cookie for authentication purposes. This particular code example is insecure because an attacker could possibly perform a cross-site scripting attack or sniff packets to find the user name within the cookie. If an attacker had the user name of a particular individual, they could forget their own cookie containing the user name and easily gain access to their account within the web application assuming that the application uses the cookie to identify a user.
Compliant Solution
The non compliant example above can be resolved by using the HttpSession class to store user information as opposes to cookies. Since HttpSession objects are server-side, it is impossible for an attacker to gain access to the session information directly through cross-site scripting attacks. Instead, the session id of the user is stored within the cookie as opposed to any excess or sensitive information. As a result, the attacker must first gain access to the session id and only then do they have a chance of gaining access to a user's account details.
private String login(HttpServletRequest request, HttpServletResponse response) { List<String> errors = new ArrayList<String>(); request.setAttribute("errors", errors); String username = request.getParameter("username"); String password = request.getParameter("password"); // Basic input validation if(username.matches("[\\w]*")) errors.add("Incorrect username format."); if(password.matches("[\\w]*")) errors.add("Incorrect password format."); if(errors.size() > 0) return "error.jsp"; UserBean dbUser = this.userDAO.lookup(username); if(!dbUser.checkPassword(password)) { errors.add("Passwords do not match."); return "error.jsp"; } HttpSession session = request.getSession(); session.invalidate(); // Invalidate old session id session = request.getSession(true); // Generate new session id session.setMaxInactiveInterval(2*60*60); // Set session timeout to two hours session.setAttribute("user", dbUser); // Store user bean within the session return "welcome.jsp"; }
In the above solution, we have switched from a cookie to a session to store user information. Additionally, the current session is invalidated and a new session is created in order to avoid session fixation attacks as noted by The Open Web Application Security Project (OWASP 2009). The timeout of the session has also been set to two hours to minimize the chance that an attacker can perform session hijacking attacks.
Risk Assessment
Noncompliance may lead to sensitive information being stored within a cookie, which may be accessible via packet sniffing or cross-site scripting attacks.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
FIO14-J |
high |
probable |
medium |
P12 |
L1 |
Bibliography
[OWASP 2009] Session Fixation in Java
[Oracle 2010] javax.servlet.http Package API