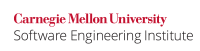
A Boxing conversion converts the values of a primitive type to the corresponding values of the reference type, for instance, from int
to the type Integer
[JLS 5.1.7 Boxing Conversion]. It can be convenient in many cases where an object parameter is desired, such as with collection classes like
Map
and List
. Another use case is to pass object references to methods, as opposed to primitive types that are always passed by value. The resulting wrapper types also help reduce clutter in code.
Noncompliant Code Example
This noncompliant code snippet [[Techtalk 07]] prints 100
as the size of the HashSet
while it is expected to print 1
. The combination of a short
and an integer
value in the operation i-1
leads to autoboxing into an Integer
object. The HashSet
contains only short
values whereas (distinctly typed) Integer
objects are being removed successively. The remove operation is as a result equivalent to a No Operation (NOP). The compiler enforces type checking so that only short
values are inserted, however, a programmer is free to remove an object of any type without triggering any exceptions since Collections<E>.remove
takes an Object parameter and not E
. Such behavior can result in unintended object retention or memory leaks.
public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for(short i=0; i<100;i++) { s.add(i); s.remove(i - 1); } System.out.println(s.size()); } }
Compliant Solution
Avoid mixing different types together with an integer
type. If inevitable, cast the autoboxed Integer
object to a Short
to remedy the issue described in the noncompliant code.
public class ShortSet { public static void main(String[] args) { HashSet<Short> s = new HashSet<Short>(); for(short i=0; i<100;i++) { s.add(i); s.remove((short)(i-1)); //cast to short } System.out.println(s.size()); } }
Risk Assessment
Numeric promotion and autoboxing while removing elements from a Collection
, can make operations on the Collection
fail silently.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP05- J |
low |
probable |
low |
P6 |
L2 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[Core Java 04]] Chapter 5
[[JLS 05]] Section 5.1.7
[[Techtalk 07]] "The Joy of Sets"
EXP04-J. Be wary of invisible implicit casts when using compound assignment operators 03. Expressions (EXP) EXP06-J. Be aware of the short-circuit behavior of the conditional AND and OR operators