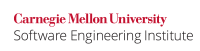
The conditional operator ?:
uses the boolean
value of one expression to decide which of the other two expressions should be evaluated [[JLS 2005]]. The conditional operator is syntactically right-associative. For instance a?b:c?d:e?f:g
is equivalent to a?b:(c?d:(e?f:g))
.
The general form of a Java conditional expression is operand1 ? operand2 : operand3
.
- If the value of the first operand (
operand1
) istrue
, then the second operand expression (operand2
) is chosen - If the value of the first operand is
false
, then the third operand expression (operand3
) is chosen
The guidelines (tabulated below) used by a Java compiler to determine the type of the result of a conditional expression are quite complicated and may result in unexpected type conversions. The first matching guideline, starting from the top of the table, is applied. In the table, *
refers to constant expressions of type int
(such as '0' or variables declared final
), Operand 2 refers to operand2
in the general form of a Java conditional given above, and Operand 3 refers to operand3
:
Operand 2 |
Operand 3 |
Resultant type |
---|---|---|
type T |
type T |
type T |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
other numeric |
other numeric |
promoted type of the 2nd and 3rd operands |
T1 = boxing conversion (S1) |
T2 = boxing conversion(S2) |
apply capture conversion to lub(T1,T2) |
Because of the complicated nature of the rules used to determine the result type of a conditional expression and the possibility of unintended type casting, it is recommended that the second and third operands of the conditional expression should always have the same type. This also applies to boxed primitives.
Noncompliant Code Example
This noncompliant code example prints the value of alpha
as A
, which is of the char
type. The third operand '0', is a constant expression of type int
whose value can be represented as a char
and hence does not cause any numeric promotion. However, this behavior depends on the value of the constant integer expression. Changing the value of the constant integer expression may lead to different behavior, as will be demonstrated in the second noncompliant code example.
public class Expr { public static void main(String[] args) { char alpha = 'A'; System.out.print(true ? alpha : 0); } }
Compliant Solution
This compliant solution recommends the use of the same types for the second and third operands of the conditional expressions. The clearer semantics help avoid confusion.
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Cast 0 as a char to explicitly state that the type of the // conditional expression should be char. System.out.print(true ? alpha : ((char) 0)); } }
Noncompliant Code Example
This noncompliant example prints 65
instead of the expected A
. 65
is the ASCII equivalent of A
. This happens because of the numeric promotion of the second operand alpha
to an int
. The numeric promotion occurs because the third operand (the constant expression '12345') is of type int
and consequently, inappropriate for being represented as a char
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; System.out.print(true ? alpha : 12345); } }
Compliant Solution
The compliant solution casts alpha
to int
for explicitly stating the result type (int
) of the conditional expression. While casting 12345
to type char
ensures that both operands in the conditional expression have the same type (and result in A
being printed), it results in data loss when an integer larger than Character.MAX_VAUE
is downsized to a char
. This compliant solution casts alpha
to int
, the wider of the operand types, to avoid this issue.
public class Expr { public static void main(String[] args) { char alpha = 'A'; // Cast alpha as an int to explicitly state that the type of the // conditional expression should be int. System.out.print(true ? ((int) alpha) : 12345); } }
Noncompliant Code Example
This noncompliant code example prints 65
instead of A
. This is because of numeric promotion of the second operand alpha
to an int
, which happens because the third operand, variable i
, is an int
.
public class Expr { public static void main(String[] args) { char alpha = 'A'; int i = 0; System.out.print(true ? alpha : i); } }
Compliant Solution
This compliant solution declares i
as a char
, ensuring that the second and third operands of the conditional expression have the same type.
public class Expr { public static void main(String[] args) { char alpha = 'A'; char i = 0; //declare as char System.out.print(true ? alpha : i); } }
Noncompliant Code Example
This noncompliant code example uses boxed and unboxed primitives of different types in the conditional expression. Consequently, the Integer
object is auto-unboxed to its primitive type int
and coerced to the primitive float
. This results in loss of precision. [[Findbugs 2008]] (sic)
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; float f = 0; System.out.print(true ? i : f); } }
Compliant Solution
This compliant solution declares both the operands as Integer
.
public class Expr { public static void main(String[] args) { Integer i = Integer.MAX_VALUE; Integer f = 0; //declare as Integer System.out.print(true ? i : f); } }
Risk Assessment
If the types of the second and third operands in a conditional expression are not the same then the result of the conditional expression may be unexpected.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP14-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[JLS 2005]] Section 15.25, Conditional Operator ? :
[[Bloch 2005]] Puzzle 8: Dos Equis
[[Findbugs 2008]] "Bx: Primitive value is unboxed and coerced for ternary operator"
EXP13-J. Remember to actually use the symbolic constants you define 04. Expressions (EXP) 05. Scope (SCP)