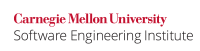
Method overloading allows one of two or more methods with the same name to be invoked, depending on the types of their arguments. The compiler inspects a call to an overloaded method and, using the declared types of the method parameters, decides which method to use. Consequently overloaded methods are quite useful. In some cases, however, ambiguity may arise because of the presence of relatively newer language features such as autoboxing and generics.
Furthermore, methods with the same parameter types that just differ in their declaration order, are typically not flagged by Java compilers. This can be potentially error prone if a developer does not consult the documentation at every use of the method. A related pitfall is to associate differing semantics with each of the overloaded methods. Defining different semantics sometimes necessitates different orderings of the same method parameters, creating a vicious circle. Consider for example a getDistance()
method whose one overloading returns the distance traveled from the source while another (with rearranged parameters) returns the uncovered distance to the destination. An implementer may not realize the difference unless the documentation is consulted at every use.
This recommendation also applies to constructors as they can also be overloaded.
Noncompliant Code Example
Notably, constructors cannot be overridden and can only be overloaded. Failure to exercise caution while passing arguments to them can create confusion as two overloadings can contain the same number of similarly typed parameters. This noncompliant code example shows the constructor Con
with three overloadings Con(int, String)
, Con(String, int)
and Con(Integer, String)
. Overloading should also be avoided when the overloaded methods provide inconsistent functionality for arguments of the same types, differing just in their declaration order. This noncompliant code example violates both these conditions.
class Con { public Con(int i, String s) { /* Initialization Sequence #1 */ } public Con(String s, int i) { /* Initialization Sequence #2 */ } public Con(Integer i, String s) { /* Initialization Sequence #3 */ } }
Compliant Solution
To be compliant, prefer the use of public static
factory methods over public
class constructors.
public static void ConName1(int i, String s) { /* Initialization Sequence #1 */ } public static void ConName2(String s, int i) { /* Initialization Sequence #2 */ } public static void ConName3(Integer i, String s) { /* Initialization Sequence #3 */ }
Noncompliant Code Example
This program provides another concrete example of noncompliance. The InformationLeak
class holds a HashMap
instance and returns a particular record to the caller based on either its key value in the map or the actual mapped value. The getData()
method has been overloaded to return the contained data indexed by the key value in one case, whereas in the other, it checks whether a particular record exists before formatting and returning it as a String
object. The InformationLeak
class inherits from java.util.HashMap
and overrides its get()
method to provide the checking functionality. This implementation can be extremely confusing to the client who will expect the getData()
methods to behave identically and not variably, depending on whether an index of the record is specified or the retrievable value.
Worse, in the presence of autoboxing, an innocent int
argument may end up invoking the undesired overloading for Integer
. This can happen if the overloading with the primitive int
type is added to a class at a later date. Clients who expect the getData()
method to fetch data based on its value will suddenly start invoking the new overloading whenever an int
argument is passed and proceed to return the record by index. This happens because a primitive argument becomes more specific in the new version whereas in the old one, autoboxing allows the selection of the method with the Integer
type parameter when an int
is passed.
class BadOverloading extends HashMap { HashMap<Integer,Integer> hm; public BadOverloading() { hm = new HashMap<Integer, Integer>(); hm.put(1, 111990000); hm.put(2, 222990000); hm.put(3, 333990000); // ssn records } public Integer getData(int i) { // Overloading sequence #1 return hm.get(i); // get record at position 'i' } public String getData(Integer i) { // Overloading sequence #2 String s = get(i).toString(); // get a particular record return (s.substring(0, 3) + "-" + s.substring(3, 5) + "-" + s.substring(5, 9)); } @Override public Integer get(Object data) { // Checks whether the ssn exists // SecurityManagerCheck() for (Map.Entry<Integer, Integer> entry : hm.entrySet()) { if(entry.getValue().compareTo((Integer)data) == 0) return entry.getValue(); // Exists } return null; } public static void main(String[] args) { BadOverloading bo = new BadOverloading(); System.out.println(bo.getData(3)); // Get record at index '3' System.out.println(bo.getData((Integer)111990000)); // Get record containing data '111990000' } }
Although the client will deduce such behavior sooner or later, other cases such as with the List
interface may go unnoticed as Bloch [[Bloch 08]] describes:
... the
List<E>
interface has two overloadings of the remove method:remove(E)
andremove(int)
. Prior to release 1.5 when it was "generified", theList
interface had aremove(Object)
method in place ofremove(E)
, and the corresponding parameter types,Object
andint
, were radically different. But in the presence of generics and autoboxing, the two parameter types are no longer radically different.
Compliant Solution
Naming the two related methods differently disqualifies the overloading and is highly recommended in this case.
public Integer getDataByIndex(int i) { /* overloading sequence #1 */ } public String getDataByValue(Integer i) { /* overloading sequence #2 */ }
Risk Assessment
Ambiguous uses of overloading can lead to unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET02- J |
low |
unlikely |
high |
P1 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Interface Collection
[[Bloch 08]] Item 41: Use overloading judiciously
MET00-J. Follow good design principles while defining methods 12. Methods (MET) MET03-J. For methods that return an array or collection prefer returning an empty array or collection over a null value