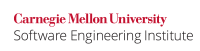
Literals that describe mathematical constants are often employed to represent well established constants. This eliminates the need to use their actual values throughout the source code and reduces the possibility of committing frivolous errors. (See DCL02-J. Use meaningful symbolic constants to represent literal values in program logic for more information)
If a mathematical constant is not declared static
, every instance of the class object will needlessly retain its own copy of the constant. Moreover, failing to declare a constant field final
can be counterproductive as highlighted in OBJ31-J. Do not use public static non-final variables. Disregarding this advice can expose the constants to pernicious thread safety issues.
At the same time, the use of static-final
modifiers should not be abused. According to the Java Language Specification [[JLS 05]] section 13.4.9 "final
Fields and Constants":
Other than for true mathematical constants, we recommend that source code make very sparing use of class variables that are declared
static
andfinal
. If the read-only nature offinal
is required, a better choice is to declare aprivate static
variable and a suitable accessor method to get its value.
Furthermore, when read only access is required, it recommends:
private static int N; public static int getN() { return N; }
instead of:
public static final int N = ...;
Another pitfall arises when static-final
is inappropriately used to declare mutable data. (See OBJ03-J. Be aware that a final reference may not always refer to immutable data).
Noncompliant Code Example
This noncompliant code example does not qualify the constant value googol (10 raised to the power 100) with the static
and final
modifiers.
public BigDecimal googol = BigDecimal.TEN.pow(100); // mathematical constant
Compliant Solution
To be compliant, ensure that all mathematical constants are declared as static-final
.
public static final BigDecimal googol = BigDecimal.TEN.pow(100);
Compliant Solution
This compliant solution ensures that all mathematical constants are declared as static-final
. Additionally, it provides read-only access to the constant by reducing its accessibility to private
and providing an accessor method.
private static final BigDecimal googol = BigDecimal.TEN.pow(100); public static BigDecimal getGoogol() { return googol; }
Exceptions
DCL31-J:EX1: According to the Java Language Specification [[JLS 05]], section 9.3 "Field (Constant) Declarations": "Every field declaration in the body of an interface is implicitly public
, static
, and final
. It is permitted to redundantly specify any or all of these modifiers for such fields."
Risk Assessment
Failing to declare mathematical constants static
and final
can lead to thread safety issues as well as inconsistent behavior.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL31- J |
low |
probable |
high |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
TODO
References
[[JLS 05]] "13.4.9 final Fields and Constants", "9.3 Field (Constant) Declarations", "4.12.4 final Variables", "8.3.1.1 static Fields"
DCL30-J. Do not attempt to assign to the loop variable in an enhanced for loop 03. Declarations and Initialization (DCL) 04. Expressions (EXP)