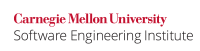
According to [[JLS 05]] Section 11.2:
"The unchecked exceptions classes are the class RuntimeException and its subclasses, and the class Error and its subclasses. All other exception classes are checked exception classes."
Unchecked exception classes such as Error
and its subclasses do not undergo compile time checking as it is tedious to account for all cases and recovery is generally difficult. However, most often recovery is not impossible or at least a graceful exit that logs the error is feasible.
Noncompliant Code Example
This code generates a StackOverflowError
due to infinite recursion. This would tend to exhaust the existing stack space.
public class StackOverflow { public static void main(String[] args) { infiniteRun(); System.out.println("Continuing..."); } private static void infiniteRun() { infiniteRun(); } }
Compliant Solution
This compliant solution shows how a try-catch
block can be used to capture java.lang.Error
or java.lang.Throwable
. A log entry can be made at this point followed by attempts to free system resources.
public class StackOverflow { public static void main(String[] args) { try { infiniteRun(); }catch(Throwable t) { System.out.println("Handling error..."); //free cache, release resources and log error to file } System.out.println("Continuing..."); } private static void infiniteRun() { infiniteRun(); } }
Note that this solution is an exception to EXC32-J. Do not catch RuntimeException since it catches Throwable
in an attempt to handle the error.
Risk Assessment
Allowing a system error to propagate right out of a Java program may result in a denial-of-service attack.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXC03-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
Other Languages
This rule appears in the C++ Secure Coding Standard as ERR30-CPP. Try to recover gracefully from unexpected errors.
References
[[JLS 05]] Section 11.2, Compile-Time Checking of Exceptions
[[Kalinovsky 04]] Chapter 16, Intercepting Control Flow - Intercepting System Errors
EXC02-J. Prevent exceptions while logging data 11. Exceptional Behavior (EXC) EXC04-J. Prevent against inadvertent calls to System.exit() or forced shutdown