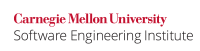
An attacker can bypass security checks if defensive copies of untrusted method parameters are made and security decisions are based on these copies. An example of an untrusted method argument is an object instance whose class provides a clone()
method and the class itself is non-final.
Noncompliant Code Example
This noncompliant code example defines a validateValue()
method that validates a time value.
private Boolean validateValue(long time) { // Perform validation return true; // If the time is valid } private void storeDateinDB(java.util.Date date) throws SQLException { final java.util.Date copy = (java.util.Date)date.clone(); if (validateValue(copy.getTime())) { Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... } }
The storeDateinDB()
method accepts an untrusted date
argument and creates a copy using the clone()
method. The attacker can override the getTime()
method so that it passes validation when called for the first time, but provides an unexpected value when it is used a second time.
public class MaliciousDate extends java.util.Date { private static int count = 0; @Override public long getTime() { java.util.Date d = new java.util.Date(); return (count++ == 1) ? d.getTime() : d.getTime() - 1000; } }
Compliant Solution
This compliant solution creates a new java.util.Date
object which is subsequently used for access control checks and insertion into the database.
private void storeDateinDB(void) throws SQLException { final java.util.Date copy = new java.util.Date(date.getTime()); if (validateValue(copy.getTime())) { Connection con = DriverManager.getConnection("jdbc:microsoft:sqlserver://<HOST>:1433","<UID>","<PWD>"); PreparedStatement pstmt = con.prepareStatement("UPDATE ACCESSDB SET TIME = ?"); pstmt.setLong(1, copy.getTime()); // ... } }
Risk Assessment
Using the clone()
method to copy untrusted argument can invalidate security checks.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET08-J |
high |
likely |
low |
P27 |
L1 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Sterbenz 2006]]
MET07-J. Do not invoke overridable methods on the clone under construction 05. Methods (MET) MET09-J. Always provide feedback about the resulting value of a method