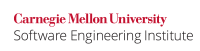
Unlike method overriding, in method overloading the choice of which method to invoke is determined at compile time. Even if the runtime type differs for each invocation, in overloading, the method invocations depend on the type of the object at compile time.
Noncompliant Code Example
This noncompliant example shows how the programmer can confuse overloading with overriding. At compile time, the type of the object array is Collection
. The messages that one would typically expect are Set invoked
, ArrayList invoked
and Collection is not recognized
. However, in all three instances Collection is not recognized
gets displayed. This is because in overloading, the method invocations are not affected by the runtime types but only the compile time type (Collection
). It is dangerous to implement overloading to tally with overriding, more so, because the latter is characterized by inheritance unlike the former.
import java.util.Set; import java.util.ArrayList; import java.util.Collection; import java.util.HashSet; import java.util.TreeSet; public class Overloader { public static String display(Set s) { return "Set invoked"; } public static String display(ArrayList l) { return "ArrayList invoked"; } public static String display(Collection c) { return "Collection is not recognized"; } public static void main(String[] args) { Collection[] invokeAll = new Collection[] {new HashSet(), new ArrayList(), new TreeSet()}; for (int i = 0; i < invokeAll.length; i++) System.out.println(display(invokeAll[i])); } }
Compliant Solution
This compliant solution uses a single display
method and instanceof
to distinguish between different types. The output is Set invoked, ArrayList invoked, Set invoked
which is expected. Do not create ambiguity while using overloading so that the code is clean and easy to understand.
import java.util.Set; import java.util.ArrayList; import java.util.Collection; import java.util.HashSet; import java.util.TreeSet; public class Overloader { public static String display(Collection c) { return (c instanceof Set ? "Set invoked" : (c instanceof ArrayList ? "ArrayList invoked" : "Collection is not recognized")); } public static void main(String[] args) { Collection[] invokeAll = new Collection[] {new HashSet(), new ArrayList(), new TreeSet()}; for (int i = 0; i < invokeAll.length; i++) System.out.println(display(invokeAll[i])); } }
Risk Assessment
Ambiguous uses of overloading can lead to unexpected results.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
MET02-J |
low |
unlikely |
high |
P1 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] Interface Collection
[[Bloch 08]] Item 41: Use overloading judiciously
MET01-J. Follow good design principles while defining methods 09. Methods (MET) MET03-J. For methods that return an array or collection prefer returning an empty array or collection over a null value