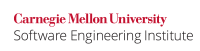
Null pointer dereferencing occurs when a null
variable is treated as if it were a valid object or field and is used without checking its state. This condition results in a NullPointerException
, which could result in denial of service. For additional information, see the related guideline "EXC15-J. Do not catch NullPointerException."
Noncompliant Code Example
This noncompliant example shows a bug in Tomcat version 4.1.24, initially discovered by Reasoning [[Reasoning 2003]]. The cardinality
method was designed to return the number of occurrences of object obj
in collection col
. One valid use of the cardinality
method is to determine how many objects in the collection are null
. However, because membership in the collection is checked with the expression obj.equals(elt)
, a null pointer dereference is guaranteed whenever obj
is null
.
public static int cardinality(Object obj, final Collection col) { int count = 0; Iterator it = col.iterator(); while (it.hasNext()) { Object elt = it.next(); if ((null == obj && null == elt) || obj.equals(elt)) { // null pointer dereference count++; } } return count; }
Compliant Solution
This compliant solution eliminates the null
pointer dereference.
public static int cardinality(Object obj, final Collection col) { int count = 0; Iterator it = col.iterator(); while (it.hasNext()) { Object elt = it.next(); if ((null == obj && null == elt) || (null != obj && obj.equals(elt))) { count++; } } return count; }
Automated Detection
Null pointer dereferences can happen in path-dependent ways. Limitations of automatic detection tools can require manual inspection of code [[Hovemeyer 2007]] to detect instances of null pointer dereferences. Annotations for method parameters that must be non-null can reduce the need for manual inspection by assisting automated null pointer dereference detection.
Risk Assessment
Dereferencing a null
pointer can lead to a denial of service. For example, Java Web Start applications and applets particular to JDK version 1.6, prior to update 4, were affected by a bug that had some noteworthy security consequences. A NullPointerException
was generated in some isolated cases when the application or applet attempted to establish an HTTPS connection with a server [[SDN 2008]]. The failure to establish a secure HTTPS connection with the server caused a denial of service: clients were temporarily forced to use an insecure http channel for data exchange. In multithreaded programs, null pointer dereferences can violate cache coherency policies and can cause resource leaks.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP11-J |
low |
likely |
high |
P3 |
L3 |
Automated Detection
The Coverity Prevent Version 5.0 FORWARD_NULL checker can detect the instance where reference is checked against null but then dereferenced anyway.
Related Guidelines
MITRE CWE: CWE-479
CERT C Secure Coding Standard: "EXP34-C. Do not dereference null pointers"
CERT C++ Secure Coding Standard: "EXP34-CPP. Ensure a null pointer is not dereferenced"
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="fd08d648-8242-4f30-a47f-aeae6343150b"><ac:plain-text-body><![CDATA[ |
[[API 2006 |
AA. Bibliography#API 06]] |
[method doPrivileged() |
http://java.sun.com/javase/6/docs/api/java/security/AccessController.html#doPrivileged(java.security.PrivilegedAction)] |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="5b6b1abf-ea39-419a-9744-d5027b6e66c4"><ac:plain-text-body><![CDATA[ |
[[Hovemeyer 2007 |
AA. Bibliography#Hovemeyer 07]] |
|
]]></ac:plain-text-body></ac:structured-macro> |
|
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="f2ccb3bd-015e-49c8-8ac6-1b53a79abbb6"><ac:plain-text-body><![CDATA[ |
[[Reasoning 2003 |
AA. Bibliography#Reasoning 03]] |
Defect ID 00-0001 |
]]></ac:plain-text-body></ac:structured-macro> |
|
|
Null Pointer Dereference |
||||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="fbfcb363-bfb5-477d-be38-6c554d682ca6"><ac:plain-text-body><![CDATA[ |
[[SDN 2008 |
AA. Bibliography#SDN 08]] |
[Bug ID 6514454 |
http://bugs.sun.com/bugdatabase/view_bug.do?bug_id=6514454] |
]]></ac:plain-text-body></ac:structured-macro> |
EXP10-J. Ensure that autoboxed values have the intended type 02. Expressions (EXP) EXP12-J. Use the same type for the second and third operands in conditional expressions