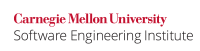
Java 1.5 supports use of enumerated types, these enums look just like their C, C++, and C# counterparts. But, in Java programming language enums are far more powerful than their counterparts in other languages, which are little more than glorified integers. All enums have an ordinal
method, which returns the numerical position of each enum constant in its type.
Java Language Specification, in Section 8.9, "Enums" does not specify the use of ordinal()
in programs. Improper use of ordinal()
method in program logic can cause errors in programs.
In Javadoc, use of ordinal() is defined as
public final int ordinal()
returns the ordinal of the enumeration constant (its position in its enum declaration, where the initial constant is assigned an ordinal of zero). Most programmers will have no use for this method. It is designed for use by sophisticated enum-based data structures, such as
EnumSet
andEnumMap
.
Although, it is clearly defined as a helper function to EnumSet
and EnumMap
, poor understanding of ordinal
generally causes errors in the programs.
Noncompliant Code Example (Using ordinal as a enum attribute)
This noncomplaint code example declares enum HydroCarbons
and uses its ordinal()
method to find the attribute, numberOfCarbons
, of enum constants.
public enum HydroCarbons { METHANE, ETHANE, PROPANE, BUTANE, PENTANE, HEXANE, HEPTANE, OCTANE, NONANE, DECANE; public int getNumberOfCarbons() { return ordinal() + 1; } } public class TestHC { public static void main(String args[]) { ... HydroCarbons hc = HydroCarbons.HEXANE; int index = hc.getNumberOfCarbons(); int noHyd = NumberOfHydrogen[index]; // Can cause ArrayIndexOutOfBoundsException } }
While the enum code above generally works, its maintenance is susceptible to vulnerabilities. If the enum constants are reordered, getNumberOfCarbon method does not return right values. Also, if we add BENZENE
(number of carbons = 6) to the enum, it is not clear where it needs to be added and causes more errors in the program.
Compliant Solution
In this compliant solution, we explicitly associate enum constants with corresponding integer values.
public enum HydroCarbons { METHANE(1), ETHANE(2), PROPANE(3), BUTANE(4), PENTANE(5), HEXANE(6), BENZENE(6), HEPTANE(7), OCTANE(8), NONANE(9), DECANE(10); private final int numberOfCarbons; HydroCarbons(int carbons) { this.numberOfCarbons = carbons; } public int getNumberOfCarbons() { return numberOfCarbons; } }
Risk Assessment
Use of ordinals to derive integer values reduces program's maintainability and leads to errors in the program.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL11-J |
low |
probable |
medium |
P4 |
L3 |
Bibliography
[[JLS 2005]] Section 8.9, "Enums"